This is a very simple solution if your images are really as you say:
#!/usr/bin/env python3
import cv2
import numpy as np
# Step 1: Load image as greyscale and invert so black is white
im = ~ cv2.imread('5DOwq.png', cv2.IMREAD_GRAYSCALE)
# Step 2: Sum across the rows left to right - black rows will sum to zero...
rowSums = np.sum(im, axis=1)
# Step 3: Find first row in image that doesn't sum to zero, i.e. first row with white pixels
row = np.nonzero(rowSums)[0][0]
# Get middle of all non-zero elements in top row
col = int(np.nonzero(im[row])[0].mean())
print(row,col)
128,283
Graphically, this is what we are doing:

Visualisation of the results at each step of the way...
Step 1:
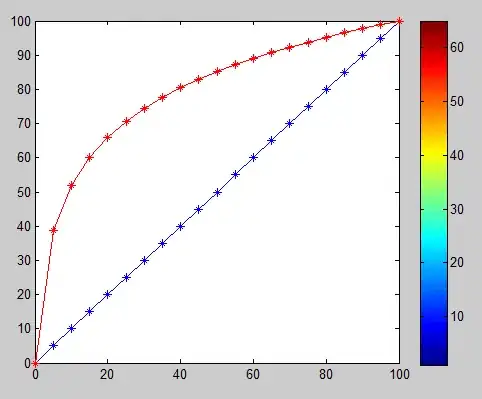
Step 2: rowSums
array([ 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 18360, 32640, 41565, 49215, 55845, 43095, 33660,
29580, 27030, 24225, 21930, 20655, 19380, 17850, 17340, 15810,
15300, 14790, 13770, 13260, 12495, 12240, 11730, 11220, 10710,
10200, 9690, 9690, 9180, 8670, 8670, 7905, 7650, 7650,
7140, 7140, 6885, 6375, 5355, 4845, 4080, 3315, 2550,
2550, 2040, 1785, 1275, 765, 510, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 510, 765, 1275, 1530, 2040, 2550, 2550, 3315,
3825, 4845, 5355, 6375, 6630, 7140, 7140, 7650, 7650,
8160, 8160, 8670, 9180, 9435, 10200, 10200, 10710, 11220,
11730, 12240, 12750, 12750, 13770, 14280, 15300, 15810, 16830,
17850, 18870, 20910, 22440, 23970, 26520, 29580, 33915, 43350,
56355, 49725, 42075, 32895, 18615, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0], dtype=uint64)
Step 3: np.nonzero(rowSums)[0]
array([128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140,
141, 142, 143, 144, 145, 146, 147, 148, 149, 150, 151, 152, 153,
154, 155, 156, 157, 158, 159, 160, 161, 162, 163, 164, 165, 166,
167, 168, 169, 170, 171, 172, 173, 174, 175, 176, 208, 209, 210,
211, 212, 213, 214, 215, 216, 217, 218, 219, 220, 221, 222, 223,
224, 225, 226, 227, 228, 229, 230, 231, 232, 233, 234, 235, 236,
237, 238, 239, 240, 241, 242, 243, 244, 245, 246, 247, 248, 249,
250, 251, 252, 253, 254, 255, 256])