First, let's do a function that returns a shuffled deck of cards.
sampleDeck = function() {
c("Ace Clubs", "2 Clubs", "3 Clubs", "4 Clubs", "5 Clubs", "6 Clubs", "7 Clubs",
"8 Clubs", "9 Clubs", "10 Clubs", "Jack Clubs", "Queen Clubs", "King Clubs",
"Ace Diamonds", "2 Diamonds", "3 Diamonds", "4 Diamonds", "5 Diamonds",
"6 Diamonds", "7 Diamonds", "8 Diamonds", "9 Diamonds", "10 Diamonds",
"Jack Diamonds", "Queen Diamonds", "King Diamonds", "Ace Hearts", "2 Hearts",
"3 Hearts", "4 Hearts", "5 Hearts", "6 Hearts", "7 Hearts", "8 Hearts",
"9 Hearts", "10 Hearts", "Jack Hearts", "Queen Hearts", "King Hearts",
"Ace Spades", "2 Spades", "3 Spades", "4 Spades", "5 Spades", "6 Spades",
"7 Spades", "8 Spades", "9 Spades", "10 Spades", "Jack Spades",
"Queen Spades", "King Spades")[sample(1:52,52)]}
sampleDeck()
output
[1] "7 Clubs" "3 Spades" "2 Clubs" "8 Diamonds" "Queen Hearts" "Ace Diamonds" "5 Diamonds"
[8] "10 Hearts" "4 Hearts" "8 Clubs" "6 Hearts" "2 Spades" "8 Hearts" "8 Spades"
[15] "Jack Hearts" "10 Diamonds" "7 Hearts" "9 Clubs" "7 Diamonds" "4 Diamonds" "Queen Clubs"
[22] "4 Spades" "Jack Clubs" "9 Diamonds" "5 Clubs" "Ace Clubs" "Queen Diamonds" "9 Spades"
[29] "Ace Spades" "3 Diamonds" "6 Clubs" "Ace Hearts" "Jack Diamonds" "6 Diamonds" "2 Diamonds"
[36] "10 Clubs" "King Clubs" "King Hearts" "7 Spades" "King Spades" "Jack Spades" "2 Hearts"
[43] "5 Hearts" "9 Hearts" "4 Clubs" "10 Spades" "King Diamonds" "3 Hearts" "5 Spades"
[50] "Queen Spades" "3 Clubs" "6 Spades"
Now it is the turn of the function that will return a shuffled deck of cards and the number of cards to be dealt to get a pair.
Deal_to_the_first_pair = function(){
deck = sampleDeck()
deck_card = str_split_fixed(deck, " ", 2)[,1]
i=2
while(length(unique(deck_card[1:i]))==length(deck_card[1:i])) i=i+1
tibble(
deck = list(tibble(deck = deck)),
ncards_dealt = i
)
}
d = Deal_to_the_first_pair()
output
# A tibble: 1 x 2
deck ncards_dealt
<list> <dbl>
1 <tibble [52 x 1]> 6
As you can see, we put out 6 cards. Let's see what the cards were.
d$deck[[1]]$deck[1:d$ncards_dealt]
output
[1] "8 Clubs" "King Hearts" "5 Diamonds" "3 Hearts" "2 Clubs" "3 Spades"
As you can see we have a couple of threes
Finally, let's do 10,000 tries.
library(tidyverse)
set.seed(1111)
ndealings = 10000
df = tibble(i = 1:ndealings) %>%
mutate(data = map(i, ~Deal_to_the_first_pair())) %>%
unnest(data)
df
output
# A tibble: 10,000 x 3
i deck ncards_dealt
<int> <list> <dbl>
1 1 <tibble [52 x 1]> 7
2 2 <tibble [52 x 1]> 8
3 3 <tibble [52 x 1]> 5
4 4 <tibble [52 x 1]> 9
5 5 <tibble [52 x 1]> 5
6 6 <tibble [52 x 1]> 8
7 7 <tibble [52 x 1]> 5
8 8 <tibble [52 x 1]> 5
9 9 <tibble [52 x 1]> 3
10 10 <tibble [52 x 1]> 2
# ... with 9,990 more rows
Let us visualize the achieved result
df %>% ggplot(aes(ncards_dealt)) +
geom_boxplot()
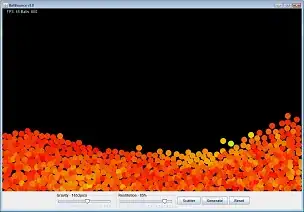
Update 1
A little update. To be fully compatible with your task, I have slightly improved the Deal_to_the_first_pair
function so that it takes a shuffled deck of cards as an argument and returns the number of cards until the first pair occurs.
Deal_to_the_first_pair2 = function(deck){
deck_card = str_split_fixed(deck, " ", 2)[,1]
i=2
while(length(unique(deck_card[1:i]))==length(deck_card[1:i])) i=i+1
i
}
set.seed(1111)
df2 = tibble(i = 1:ndealings) %>%
mutate(deck = map(i, ~sampleDeck())) %>%
mutate(ncards_dealt = map(deck, ~Deal_to_the_first_pair2(.x))) %>%
unnest(ncards_dealt)
df2
output
# A tibble: 10,000 x 3
i deck ncards_dealt
<int> <list> <dbl>
1 1 <chr [52]> 7
2 2 <chr [52]> 8
3 3 <chr [52]> 5
4 4 <chr [52]> 9
5 5 <chr [52]> 5
6 6 <chr [52]> 8
7 7 <chr [52]> 5
8 8 <chr [52]> 5
9 9 <chr [52]> 3
10 10 <chr [52]> 2
# ... with 9,990 more rows
Now you have it just like in your homework :-).