Yes, reference variables
You are correct, alex
, linda
, and john
are all reference variables.
All three are little containers that hold either nothing (null) or a reference (pointer) to an object setting in memory somewhere else. Those three variables do not hold an object, but they know how to get you to an object.
Given the syntax in Java, we tend to forget about this distinction. In our daily programming work, we usually think of alex
as the object. We generally think:
alex
holds a Person
object representing the human known as Alex
… whereas the technical truth is:
alex
may hold a reference that can lead us to the chunk of memory elsewhere holding our Person
object that we want to access
In a tutorial, it can be tiring and distracting to constantly refer to alex
and linda
as reference variables. So perhaps we should give the author of your tutorial some poetic license to call them objects.
Example code
Some example code, using the records feature in Java 16+.
record Person( String firstName , String lastName , Color favoriteColor ) { }
Person alex = new Person( "Alex" , "Peterson" , Color.MAGENTA );
Person linda = new Person( "Linda" , "Greystone" , Color.GREEN );
Person john = new Person( "John" , "Petrov" , Color.YELLOW );
Each call to new Person
instantiates an object, that is, allocates a chunk of memory somewhere in RAM to hold our three member fields (state) along with handles to the executable code we think of as named methods (behavior). (For records, the compiler implicitly creates constructor, getters, equals
& hashCode
, and toString
methods. Plus, you can add more.)
The call to new Person
returns a reference, essentially the address in memory where the new Person object/instance lives. We store that reference in the reference variable alex
or linda
. We declared those variables as being references to hold objects of type Person
.
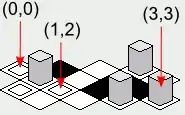