You are executing the function without any parameters, thus your 2nd line errors out as you don't have input
for it.
If you wish to hash all non-empty cells in A2:F2360
which is located in Sheet1
, you need to first access those cells, read their values, modify them using the MD5
function, and write it back again. See code below.
Script:
// function that will hash the values
function MD5 (input) {
var rawHash = Utilities.computeDigest(Utilities.DigestAlgorithm.MD5, input);
var txtHash = '';
for (i = 0; i < rawHash.length; i++) {
var hashVal = rawHash[i];
if (hashVal < 0) {
hashVal += 256;
}
if (hashVal.toString(16).length == 1) {
txtHash += '0';
}
txtHash += hashVal.toString(16);
}
return txtHash;
}
// main function that will call your MD5 function
// execute this one instead of MD5
function main() {
var ss = SpreadsheetApp.getActiveSpreadsheet();
// access the values of the sheet name indicated
var sheet = ss.getSheetByName('Sheet1');
// access the values of the range indicated
var range = sheet.getRange('A2:F2360');
// get the values in the mentioned range
var values = range.getValues();
// modify each cell to be hashed using MD5 function above.
// added a condition where it won't write anything for blank cells as MD5 still hashes blank string
var output = values.map(row => row.map(cell => cell ? MD5(cell) : cell));
// write the modified values to the same range
range.setValues(output);
}
Sample data:

Output:
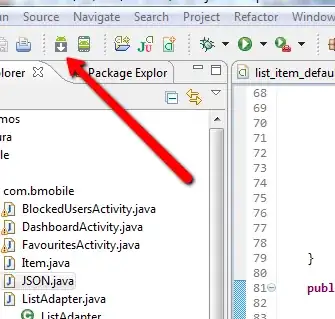
Note:
- If you are unfamiliar with Apps Script, read more about it on the references listed but not limited to:
References: