Let's imagine you've got 20 players and each one got some score.
As you decided to create an array of int
s to store all scores, so do I:
int[] scores = new int[20];
For example, I fill that array with random unique scores from 10 to 100. You fill in your way:
int[] scores = new int[20];
Random rnd = new Random();
for (int i = 0; i < scores.Length; i++)
{
int score = 0;
// This loop used to generate new random int until it wouldn't be unique (wouldn't exist in array)
while (score == 0 || scores.Contains(score))
score = rnd.Next(10, 100);
scores[i] = score;
lblAllScores.Text += "Player #" + (i + 1) + " score: " + scores[i] + "\n";
}
I append each score and Player # (as number of iteration + 1) to some Label
on Form
to show all players and their scores. Something like this:
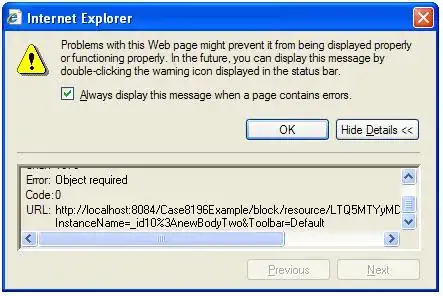
Now you (and I) want take top 10 scores (obviously in descending order, from highest to lowest). Here the keywords are Take and Descending order. In C# through System.Linq
namespace provided such pretty extension methods like Take
and OrderByDescending
. And seems they can give us desired result if we use it properly.
I (and you) creating a new array of int
s to store only Top 10 Scores. Then a take initial array scores
and use OrderByDescending
method to sort values in it from highest to lowest. (x => x)
provided as selector to determine key by which method should order values - in our case by value itself. And then I use Take(10)
method to take first 10 scored from that sorted array - this is our Top 10 Scores:
int[] top10Scores = scores.OrderByDescending(x => x).Take(10).ToArray();
And all I need to do - put this into Form
, which I can do by simple iteration on top10Scores
array with for
loop. But I (and probably you) want to know what Player has that score. Upper we use (index of array + 1) as Player Number. And we know that scores are unique for each Player. Than now we can take the score value from top10Scores
array and try to find its index in source scores
array with Array.FindIndex
method:
for (int i = 0; i < top10Scores.Length; i++)
{
int playerNumber = Array.FindIndex(scores, score => score == top10Scores[i]) + 1;
lblTop10Scores.Text += "Player #" + playerNumber + " score: " + top10Scores[i] + "\n";
}
And at our Form we have this:
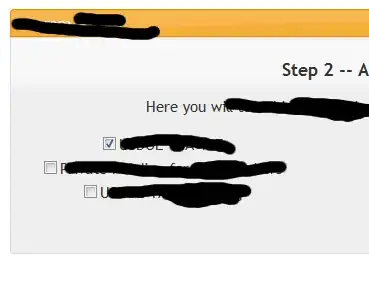
Complete code:
private void ScorePlayers()
{
int[] scores = new int[20];
Random rnd = new Random();
for (int i = 0; i < scores.Length; i++)
{
int score = 0;
while (score == 0 || scores.Contains(score))
score = rnd.Next(10, 100);
scores[i] = score;
lblAllScores.Text += "Player #" + (i + 1) + " score: " + scores[i] + "\n";
}
int[] top10Scores = scores.OrderByDescending(x => x).Take(10).ToArray();
for (int i = 0; i < top10Scores.Length; i++)
{
int playerNumber = Array.FindIndex(scores, score => score == top10Scores[i]) + 1;
lblTop10Scores.Text += "Player #" + playerNumber + " score: " + top10Scores[i] + "\n";
}
}
Please note, that this is only a sketch and example to demonstrate how to use OrderByDescending
and Take
extension methods to get first 10 elements of sorted from highest to lowest value collection. In real application you probably will have at least simple Dictionary<string, int>
, where would be stored player name (as string
) and his score (as int
). Or, more likely, it would be a model class called Player
with Name
and Score
properties and each Player
would be stored in some List<Player>
:
public class Player
{
public string Name { get; set; }
public int Score { get; set; }
}
Hope you can find your solution and this example would be helpful for you.