Example to explain comment.
You have some table of N columns and N rows:
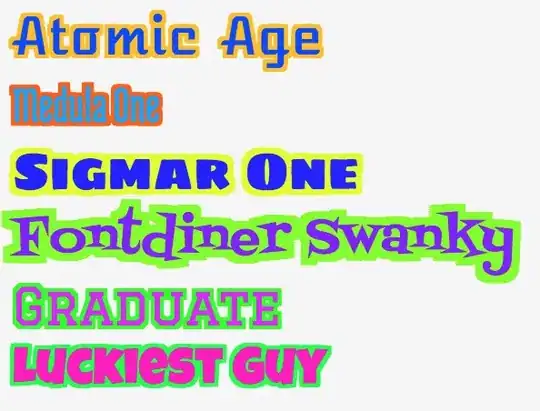
You can create a class which would represent one object (one entity):
public class Person
{
}
and fill it with Properties, which would be similar for each entity (your 30 columns):
public class Person
{
public string Name { get; set;}
public string Surname { get; set;}
public int Age { get; set;}
public string City { get; set;}
public string Gender { get; set;}
// and other of 30 columns
}
So, each row in table represents some Person (in that example). 5000 rows = we would have 5000 Persons. As they are similar entities - we can store them as collection or Persons. We can use (for simple example):
Person[]
- array of Persons;
List<Person>
- list of Persons;
Let's imagine, how to read data from table and create a List of Persons from it.
public void ReadTable(Table myTable) // We have Table with Rows and Columns
{
// Initializing our collection of Persons
List<Person> persons = new List<Person>;
// We need to read all 5000 rows, so iterating them
for (int i = 1; i <= myTable.Rows.Count; i++) // myTable.Rows.Count = 5000
{
// Creating a Person
Person person = new Person();
// Reading each cell value, accessing to it through RowIndex & ColumnIndex
// Row 1 Column 1 is Name
string name = myTable.Rows[i].Columns[1].Value.ToString(); // John
// Row 1 Column 2 is Surname
string surname = myTable.Rows[i].Columns[2].Value.ToString(); // Wick
// Row 1 Column 3 is Age
int age = int.Parse(myTable.Rows[i].Columns[3].Value); // 55
// Row 1 Column 4 is City
string city = myTable.Rows[i].Columns[4].Value.ToString(); // New York
// Row 1 Column 5 is Gender
string gender = myTable.Rows[i].Columns[5].Value.ToString(); // Male
//Received from table values we add to a Person:
person.Name = name;
person.Surname = surname;
person.Age = age;
person.City = city;
person.Gender = gender;
// And finally adding Person to a collection (list) of Persons:
persons.Add(person);
// And same would happen with other 5000 rows
}
}
So after reading you will have persons
list with 5000 items. You can manipulate them, edit, add new and remove existing - whatever.