If you really want to be able to read and write images, simply, via a FILE pointer, you'll want to look at a file format called a .ppm. (Portable Pixel Map.) This assumes, of course, that you can convert your image files to a .ppm, but that is easily achievable with the imagemagick command-line tool. You can use imagemagick to convert the .ppms you write to any output type you like.
The format is insanely simple:
- Starts with the two character (the "magic number") "P6"
- The P6 is followed by a whitespace, then the width (in ascii) of the image.
- The width is followed by a whitespace, then the height (in ascii) of the image.
- The last bit of the header is the "maximum value" of an entry. Just use 255. Follow this with a whitespace.
- After that, you just write, in binary, RGB values. One unsigned char per channel.
Example:
P6 128 128 255
[128 * 128 * 3 bytes of data go here, in row major order. The top-left
pixel is first, then the pixel to the right of it. When you get to the
end of a row, just write the first pixel of the next row. That's all
there is. No other header info, no terminators, etc.]
Example:
#include <stdio.h>
void main() {
FILE * out;
out = fopen("color_test.ppm", "wb");
fprintf(out, "P6 256 256 255\n");
for(int r=0; r<256; r++) {
for(int b=0; b<256; b++) {
fputc(r, out);
fputc(0, out);
fputc(b, out);
}
}
fclose(out);
}
This generates:
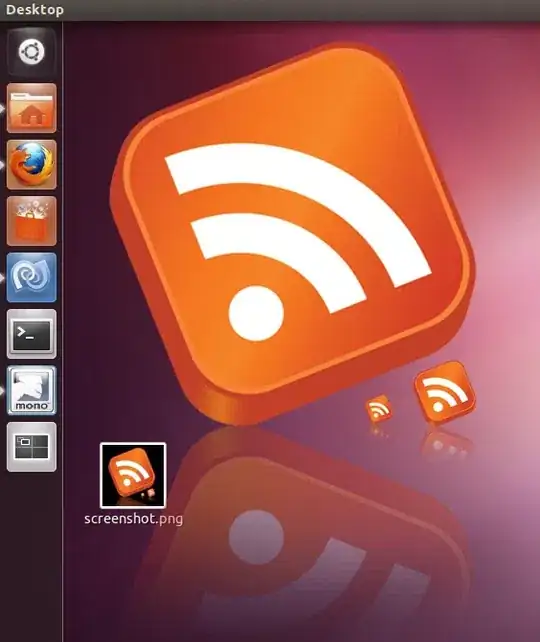
Reading files is similarly easy. You can just pull them into a 3d array of unsigned chars, manipulate them in memory however you like, then write that data back out to another file much as my example does here.