I found it, it's a library called kaptcha (Internet Archive version of the Google Code page here), which seems to be a fork of the SimpleCaptcha Java library. kaptcha was created by Jon Stevens (see here), who cloned the Google Code repo to GitHub here. GitHub user penggle has also cloned that repository and made it available on Maven.
To try it out without spinning up a huge Maven project, e.g. to generate a lot of training data for a machine learning algorithm, do the following:
- Download
kaptcha-2.3.2.zip
from kaptcha's downloads page.
- From that ZIP file, extract
kaptcha-2.3.2.jar
and put it into a new directory.
- In the directory of the JAR file, create a new file called
Main.java
and put the following code into it. As expected, all these pages just use the default settings from the file src/java/com/google/code/kaptcha/util/Config.java
in the ZIP. Of course you can replace the use of DefaultTextCreator
(which even only uses the letters abcde2345678gfynmnpwx
by default) by any string you want.
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.Properties;
import javax.imageio.ImageIO;
import com.google.code.kaptcha.util.Config;
import com.google.code.kaptcha.impl.DefaultKaptcha;
import com.google.code.kaptcha.text.impl.DefaultTextCreator;
public class Main {
public static void main(String[] args) throws IOException {
Properties properties = new Properties();
Config config = new Config(properties);
DefaultKaptcha kaptcha = new DefaultKaptcha();
kaptcha.setConfig(config);
DefaultTextCreator textCreator = new DefaultTextCreator();
textCreator.setConfig(config);
BufferedImage image = kaptcha.createImage(textCreator.getText());
File output = new File("out.jpg");
ImageIO.write(image, "jpg", output);
}
}
- Run this Java code using the following two shell commands:
javac -cp kaptcha-2.3.2.jar Main.java
java -cp kaptcha-2.3.2.jar:. Main
- The output JPG you will get will look like this:
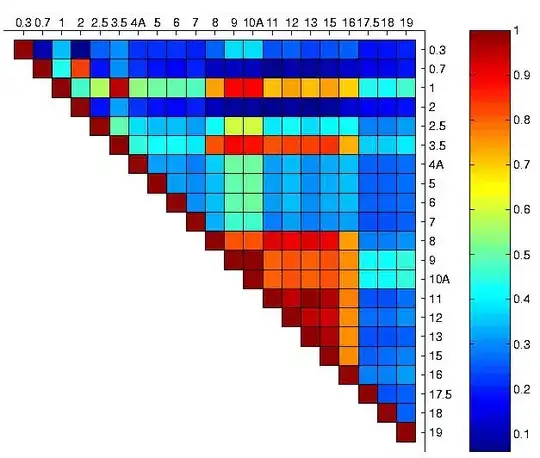
- To change the config, add the line
import com.google.code.kaptcha.Constants;
at the top of Main.java
and then, for example, add the command properties.setProperty(Constants.KAPTCHA_BORDER, "no");
before creating the Config
object:
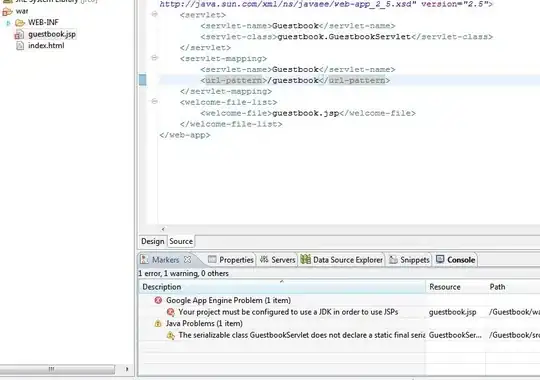