You can create a custom style to the CheckBox
and adjust the end padding with a negative value:
<style name="checkbox_style" parent="android:style/Widget.Holo.Light.CompoundButton.CheckBox">
<item name="android:paddingRight">-7dp</item>
</style>
And apply it to your app's theme:
<item name="checkboxStyle">@style/checkbox_style</item>
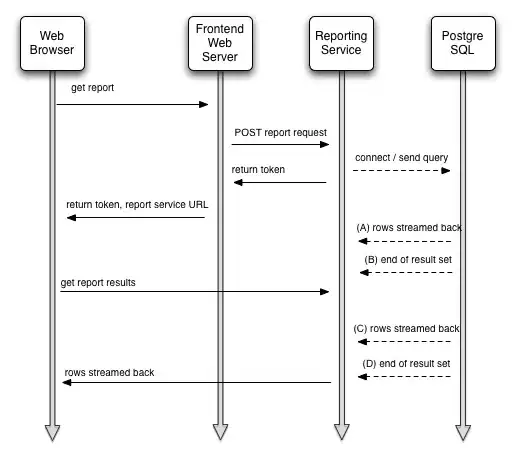
Note: By default there should be some margin surrounds a menu item; so you can't send the checkBox
to the far end, as its right edge will cut:
When using -8dp:
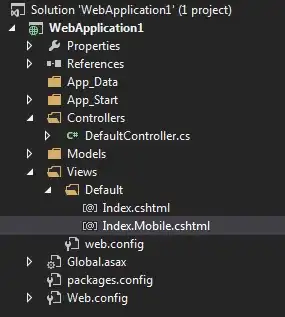
So, you need to be careful in handling this.
UPDATE
but it affects all the CheckBoxes
in my app
This requires to reverse this padding in all of CheckBoxes
in your layouts; and there are options for this:
- First option: add
android:paddingRight="7dp"
/ android:paddingEnd="7dp"
to all the CheckBoxes
.
- Second option: Create a custom
CheckBox
class and add this padding to its constructors; and use this customized CheckBox instead of the default CheckBox
:
public class MyCheckBox extends AppCompatCheckBox {
public MyCheckBox(Context context) {
super(context);
init();
}
public MyCheckBox(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
private void init() {
int px = (int) TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_DIP,
7f, // dp value
getResources().getDisplayMetrics()
);
setPadding(0, 0, px, 0);
}
public MyCheckBox(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
}
And to use it:
<!-- Add the full path of the customized CheckBox -->
<com.example.android......MyCheckBox
android:id="@+id/checkbox2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
Hint: If you target API-17+, then use android:paddingEnd
instead android:paddingRight