I have done this slightly differently and it serves the purpose. I have several classes. They extend JPanel, serve different purposes and use JDialogs to get users' inputs.
A typical class would contain necessary fields, save and cancel buttons.
public class EnterpriseGUI extends javax.swing.JPanel {...} would look like this:
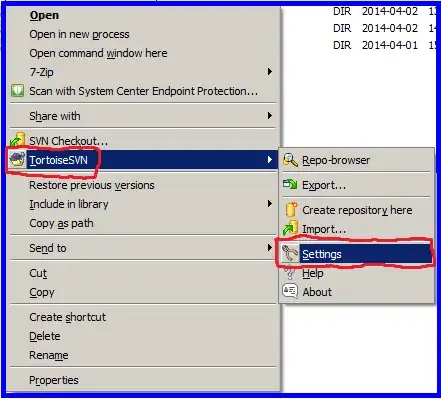
Class EnterpriseGUI has a private variable jdialog and a method to set jdialog. Also ActionEvent for 'save' button and 'cancel' button.
private JDialog jdialog;
public void setJDialog(JDialog jdialog) {
this.jdialog = jdialog;
}
private void btnSaveActionPerformed(java.awt.event.ActionEvent evt) {
// Do your stuff..
jdialog.dispose();
}
private void btnCancelActionPerformed(java.awt.event.ActionEvent evt) {
// Discard your stuff..
jdialog.dispose();
}
Finally, I create an instance of EnterpriseGUI from any other class as needed and embed it into JDialog.
Class OtherClass{
private JDialog dialog;
private EnterpriseGUI = new enterprisegui();
private void button1ActionPerformed(java.awt.event.ActionEvent evt) {
this.dialog = new JDialog(this, "My dialog", true);
this.dialog.setResizable(false);
enterprisegui.setJDialog(dialog);
this.dialog.getContentPane().add(enterprisegui);
this.dialog.pack();
Dimension Size = Toolkit.getDefaultToolkit().getScreenSize();
this.dialog.setLocation(new Double((Size.getWidth()/2) -
(dialog.getWidth()/2)).intValue(), new Double((Size.getHeight()/2) -
(dialog.getHeight()/2)).intValue());
this.dialog.setVisible(true);
}
}