If you want TextBox
with placeholder and be able customize placeholder color - you shouldn't use SendMessage
and EM_SETCUEBANNER
. They always make placeholder with default gray color.
To get desired result, you should create your own HintTextBox
(or anyway you want to name it). Example below taken from @Reza Aghaei answer (here) and edited a bit.
Code:
HintTextBox.cs
using System.Drawing;
using System.Windows.Forms;
namespace MyWinFormsApp
{
public class HintTextBox : TextBox
{
public HintTextBox()
{
Width = 150; // Some default width
Multiline = true; // Multiline by default
}
private string hint = "Input some text..."; // Default placeholder text
private Color hintColor = Color.Red; // Default placeholder color
// Set another Hint text through this property
public string Hint
{
get => hint;
set
{
hint = value;
Invalidate();
}
}
// Set placeholder color you wish through this property
public Color HintColor
{
get => hintColor;
set
{
hintColor = value;
Invalidate();
}
}
// Drawing placeholder on WM_PAINT message
protected override void WndProc(ref Message message)
{
base.WndProc(ref message);
if (message.Msg == 15) // WM_PAINT or 0xF
{
if (!Focused &&
string.IsNullOrEmpty(Text) &&
!string.IsNullOrEmpty(Hint))
{
using (Graphics g = CreateGraphics())
{
TextRenderer.DrawText(g, Hint, Font,
ClientRectangle, hintColor, BackColor,
TextFormatFlags.Top | TextFormatFlags.Left);
}
}
}
}
}
}
Usage example:
private void Form1_Load(object sender, EventArgs e)
{
// Create two HintTextBoxes with some placeholder and color
HintTextBox hintTextBox1 = new HintTextBox();
// Customize placeholder color through HintColor property
hintTextBox1.HintColor = Color.Magenta;
// Set placeholder with a text you wish
hintTextBox1.Hint = "My awesome hint";
// Some another HintTextBox
HintTextBox hintTextBox2 = new HintTextBox();
hintTextBox2.HintColor = Color.SeaGreen;
hintTextBox2.Hint = "My another hinted TextBox...";
// For example, I put both HintTextBoxes to FlowLayoutPanel
FlowLayoutPanel flowLayoutPanel = new FlowLayoutPanel();
flowLayoutPanel.Dock = DockStyle.Fill;
flowLayoutPanel.FlowDirection = FlowDirection.TopDown;
flowLayoutPanel.Controls.Add(hintTextBox1);
flowLayoutPanel.Controls.Add(hintTextBox2);
// Add FlowLayoutPanel with 2 HintTextBoxes on a Form
this.Controls.Add(flowLayoutPanel);
}
Result:
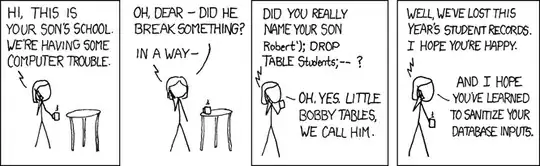