This can be achieved very simply.
First your code:
library(igraph)
actors <- data.frame(name=c("Alice", "Bob", "Cecil", "David",
"Esmeralda"))
relations <- data.frame(from=c("Bob", "Cecil", "Cecil", "David",
"David", "Esmeralda"),
to=c("Alice", "Bob", "Alice", "Alice", "Bob", "Alice"),
friendship=c(4,15,5,2,11,1))
g <- graph_from_data_frame(relations, directed=TRUE, vertices=actors)
Now let's create a graph with a thickness of 2, line color black and line type 2.
plot(g, edge.width = 2, edge.color = "black", edge.lty = 2)
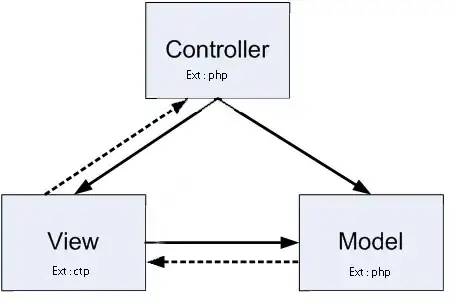
Of course, you can change it as you like
plot(g, edge.width = 5, edge.color = "black", edge.lty = 1)
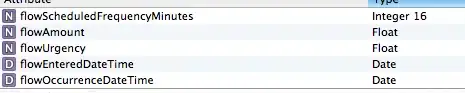
Hope that's what you meant.
Small update
Finally, you may want to find the coordinates of the elements. You can do it this way:
coords = layout_nicely(g)
coords[5,]=c(20, 20)
coords
output
[,1] [,2]
[1,] 15.21285 18.97650
[2,] 15.18511 20.08411
[3,] 14.21575 19.70269
[4,] 16.17453 19.75255
[5,] 20.00000 20.00000
Plot
plot(g, layout=coords, edge.width = 5, edge.color = "black", edge.lty = 1)
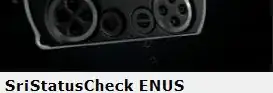
You can also set other cutout attributes
plot(g, vertex.size = 20, vertex.color = "red", vertex.shape = "square",
edge.width = 3, edge.color = "black", edge.lty = 3,
edge.label = relations$friendship, edge.curved = TRUE)
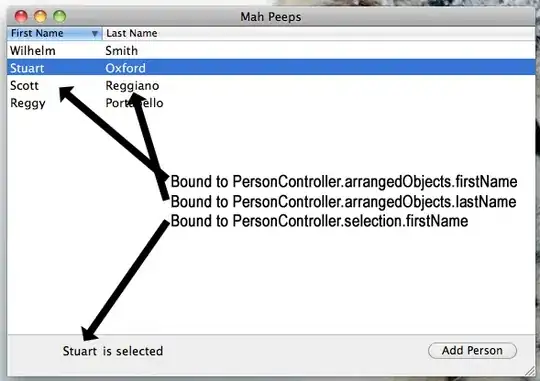
Also note that any of these parameters can also come from a vector.
So there is no obstacle for the thickness to come from the variable friendship
, for example.
plot(g, vertex.size = 20, vertex.color = "red",
vertex.shape = c("square","circle","square","circle","rectangle"),
edge.width = relations$friendship, edge.color = "black", edge.lty = c(1,2,3,1,2,3),
edge.label = 10:20, edge.curved = TRUE)
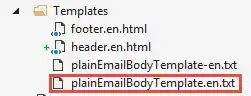