You have [an image of] a Swing GUI and you want to convert it to JavaFX.
You need a combination of layouts. For the root of the node graph, use a BorderPane
.
- The top node is a
Label
but since you want that Label
centered, you need to place it in a HBox
.
- The left node is the Bagel pane. The
RadioButton
s are placed in a VBox
.
- The center node is the Toppings pane. The
CheckBox
es are placed in a VBox
.
- The right node is the Coffee pane. The
RadioButton
s are placed in a VBox
.
- The bottom node contains the
Button
s. Since you want the Button
s centered, you need to place them in a HBox
.
The below code demonstrates. Note that the code for class BorderedTitledPane
comes from the accepted answer to this question: GroupBox / TitledBorder in JavaFX 2?
(Since JavaFX does not have a TitledBorder
as Swing does.)
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Node;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.control.Label;
import javafx.scene.control.RadioButton;
import javafx.scene.control.ToggleGroup;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.StackPane;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class OrdaCalc extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
BorderPane root = new BorderPane();
Label label = new Label("Welcome to Brandi's Bagel House");
HBox labelHBox = new HBox(label);
labelHBox.setAlignment(Pos.CENTER);
root.setTop(labelHBox);
root.setLeft(createLeftPane());
root.setCenter(createCenterPane());
root.setRight(createRightPane());
root.setBottom(createBottomPane());
Scene scene = new Scene(root);
scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm());
primaryStage.setScene(scene);
primaryStage.show();
}
private HBox createBottomPane() {
Button calculate = new Button("Calculate");
Button exit = new Button("Exit");
HBox buttonsHBox = new HBox(10.0d, calculate, exit);
buttonsHBox.setAlignment(Pos.CENTER);
return buttonsHBox;
}
private BorderedTitledPane createCenterPane() {
CheckBox creamCheese = new CheckBox("Cream Cheese");
CheckBox butter = new CheckBox("Butter");
CheckBox peachJelly = new CheckBox("Peach Jelly");
CheckBox blueberryJam = new CheckBox("Blueberry Jam");
VBox vBox = new VBox(5.0d, creamCheese, butter, peachJelly, blueberryJam);
BorderedTitledPane center = new BorderedTitledPane("Toppings", vBox);
return center;
}
private BorderedTitledPane createLeftPane() {
RadioButton white = new RadioButton("White");
RadioButton wheat = new RadioButton("Wheat");
ToggleGroup group = new ToggleGroup();
group.getToggles().addAll(white, wheat);
VBox vBox = new VBox(30.0d, white, wheat);
BorderedTitledPane left = new BorderedTitledPane("Bagel", vBox);
return left;
}
private BorderedTitledPane createRightPane() {
RadioButton none = new RadioButton("None");
RadioButton regular = new RadioButton("Regular");
RadioButton decaf = new RadioButton("Decaf");
RadioButton cappuccino = new RadioButton("Cappuccino");
ToggleGroup group = new ToggleGroup();
group.getToggles().addAll(none, regular, decaf, cappuccino);
VBox vBox = new VBox(5.0d, none, regular, decaf, cappuccino);
BorderedTitledPane right = new BorderedTitledPane("Coffee", vBox);
return right;
}
public static void main(String[] args) {
launch(args);
}
}
class BorderedTitledPane extends StackPane {
BorderedTitledPane(String titleString, Node content) {
Label title = new Label(" " + titleString + " ");
title.getStyleClass().add("bordered-titled-title");
StackPane.setAlignment(title, Pos.TOP_CENTER);
StackPane contentPane = new StackPane();
content.getStyleClass().add("bordered-titled-content");
contentPane.getChildren().add(content);
getStyleClass().add("bordered-titled-border");
getChildren().addAll(title, contentPane);
}
}
Here is file application.css
which is located in the same package as class OrdaCalc
.
.bordered-titled-title {
-fx-background-color: white;
-fx-translate-y: -16;
}
.bordered-titled-border {
-fx-content-display: top;
-fx-border-insets: 20 15 15 15;
-fx-background-color: white;
-fx-border-color: black;
-fx-border-width: 2;
}
.bordered-titled-content {
-fx-padding: 26 10 10 10;
}
Here is a screen capture.
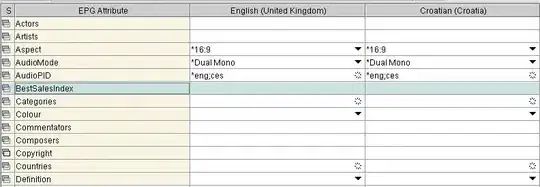