You don't only need to remove the column in the onUpgrade
method, you should also remove the column from the SQL in the onCreate
method; otherwise a new installation will have the existing STATUS column.
This then simplifies removing the column as you can then call onCreate
from the onUpgrade
method to create the table exactly as it would be for a new installation.
Note if you have any other tables then you should change the create SQL to include IF NOT EXISTS
(see below for an example).
Trying to create an existing table without IF NOT EXISTS
will result in a failure.
so :-
- Change the
onCreate
method to be
:-
@Override
public void onCreate(SQLiteDatabase db) {
String create_table = "CREATE TABLE IF NOT EXISTS " + TABLE_NAME + "( "
+ "ID INTEGER PRIMARY KEY ,"
// + STATUS + " TEXT NOT NULL, "// COMMENTED OUT
+ _ID + " TEXT NOT NULL)";
db.execSQL(create_table);
}
- adding IF NOT EXISTS isn't required but if used on any other tables (if any) then calling the
onCreate
method will not fail
- Change the
onUpgrade
step to
:-
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
if (oldVersion < 2) {
String suffix = "_old";
db.execSQL("ALTER TABLE " + TABLE_NAME + " RENAME TO " + TABLE_NAME + suffix + ";");
onCreate(db);
/* Preserves existing data */
db.execSQL("INSERT INTO " + TABLE_NAME + " SELECT ID," + _ID + " FROM " + TABLE_NAME + suffix + ";");
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME + suffix + ";");
}
}
Working Example
The following is a working example that uses your DBHelper.
MainActivity adds a row according to the database version it being :-
public class MainActivity extends AppCompatActivity {
DBHelper dbHelper;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
/* Testing */
dbHelper = new DBHelper(this);
SQLiteDatabase db = dbHelper.getWritableDatabase();
int version = db.getVersion();
ContentValues cv = new ContentValues();
cv.put(DBHelper._ID,"somedata");
if (version == 1) {
cv.put(DBHelper.STATUS,version);
}
db.insert(DBHelper.TABLE_NAME,null,cv);
}
}
Run 1
For the first run Version 1 is used and the status column is kept as per :-
public DBHelper(@Nullable Context context) {
super(context, DATABASE_NAME, null, 1);
}
@Override
public void onCreate(SQLiteDatabase db) {
String create_table = "CREATE TABLE " + TABLE_NAME + "( "
+ "ID INTEGER PRIMARY KEY ,"
+ STATUS + " TEXT NOT NULL, "
+ _ID + " TEXT NOT NULL)";
db.execSQL(create_table);
}
Using App Inspection (Database Inspector) shows :-
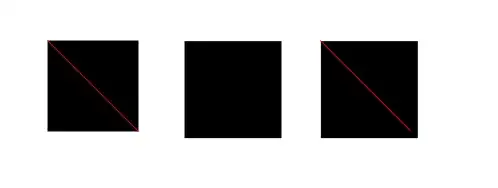
- so the database has 1 row.
Run 2
For the second run the status column is commented out and the version changed to 2 and run resulting in:-
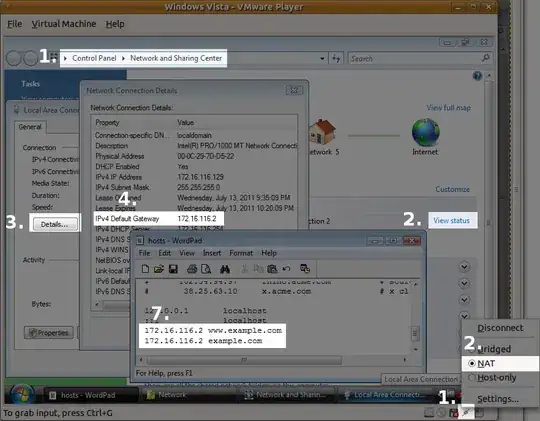
i.e. the existing data has been kept (bar the status). The table does not have the status column. Another row has been added.
Run 3
No code changes but the App was uninstalled to reflect a new installation at version 2, the result being just the 1 row added to the new version 2 table :-
