joypy.joyplot can not easily be adapted. For example, the first y-tick of each subplot is used for the name. Also, a dummy subplot is used just for the common x-axis.
An alternative could be to use Seaborn which offers easier options for customization. See for example Seaborn's ridgeplot example.
Here is a start for adapting the joyplot. (It uses seaborn's iris dataset for easier reproducibility.)
from matplotlib import pyplot as plt
from matplotlib.ticker import MaxNLocator, ScalarFormatter
import seaborn as sns # for the iris dataset
import joypy
iris = sns.load_dataset('iris')
fig, axes = joypy.joyplot(iris, by="species", column="sepal_width", hist=True, bins=20, overlap=0, grid=True,
legend=False)
for ax in axes[:-1]:
ax.set_ylabel(ax.get_yticklabels()[0].get_text())
ax.yaxis.set_major_locator(MaxNLocator())
ax.yaxis.set_major_formatter(ScalarFormatter())
fig.subplots_adjust(left=0.1)
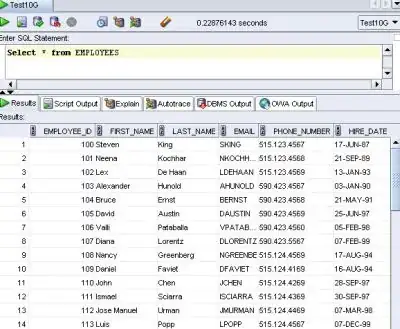
Here is a somewhat similar Seaborn plot.
from matplotlib import pyplot as plt
import seaborn as sns
iris = sns.load_dataset('iris')
sns.set_style("ticks", {'axes.grid': True})
g = sns.displot(data=iris, row='species', kind='hist', x="sepal_width", height=2, aspect=3)
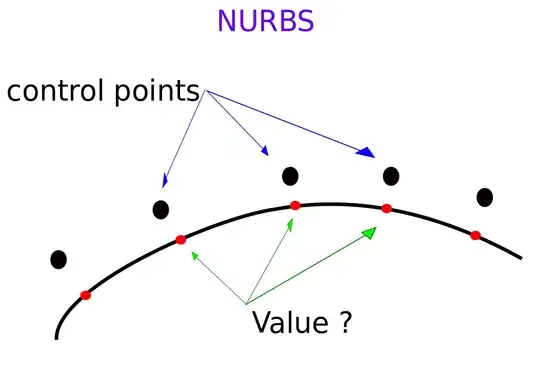