1) Circle and Text rows over an Image
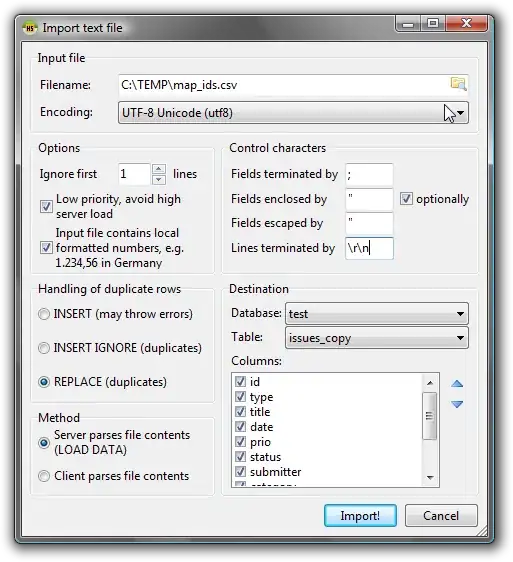
- Create a VerticalGuideline 50% from an edge
- Create an Image view
- Make a box with rounded corners to make a circle
- Constrain the circle to the VerticalGuideline
- Create a Column with your two text items
- Constrain them to the end of the circle
@Preview
@Composable
fun CircleWithTextColumnOverImage() {
ConstraintLayout(
modifier = Modifier
.background(Color.White)
.fillMaxSize()
) {
// View References
val (image, circle, textColumn) = createRefs()
// Guide line to constrain circle against
val verticalGuideline = createGuidelineFromAbsoluteLeft(.5f)
// Image
Image(
painterResource(id = R.drawable.launcher_background),
contentDescription = "Image Background",
modifier = Modifier
.size(100.dp)
.constrainAs(image) {
start.linkTo(parent.start)
end.linkTo(parent.end)
top.linkTo(parent.top)
bottom.linkTo(parent.bottom)
}
)
// Circle
Box(modifier = Modifier
.size(100.dp)
.padding(16.dp)
.background(color = Color.Red, shape = RoundedCornerShape(50.dp))
.constrainAs(circle) {
end.linkTo(verticalGuideline) // <-- Constrained to the VerticalGuideline
top.linkTo(image.top)
bottom.linkTo(image.bottom)
}
)
// Text Column
Column(modifier = Modifier
.constrainAs(textColumn) {
start.linkTo(circle.end) // <-- Constrained to the Circle
top.linkTo(circle.top)
bottom.linkTo(circle.bottom)
}) {
Text("Line 1 goes here")
Text("Line 2 goes here")
}
}
}
2) Circle and Text rows
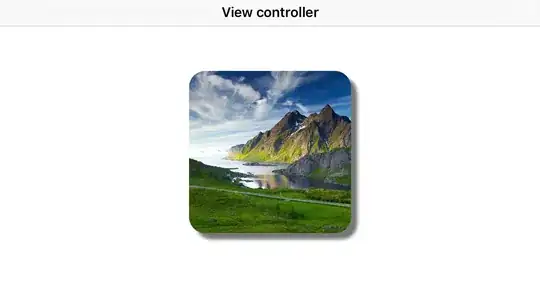
- Make a box with rounded corners to make a circle
- Create a Column with your two text items
- Constrain them together with a ConstraintLayout
@Preview
@Composable
fun CircleWithTextColumn() {
ConstraintLayout(modifier = Modifier.fillMaxSize()) {
val (circle, textColumn) = createRefs()
// Circle
Box(modifier = Modifier
.size(100.dp)
.padding(16.dp)
.background(color = Color.Red, shape = RoundedCornerShape(50.dp))
.constrainAs(circle) {
start.linkTo(parent.start)
top.linkTo(parent.top)
}
)
// Text Column
Column(modifier = Modifier
.constrainAs(textColumn) {
start.linkTo(circle.end)
top.linkTo(circle.top)
bottom.linkTo(circle.bottom)
}) {
Text("Line 1 goes here")
Text("Line 2 goes here")
}
}
}