Simple enough here is an example:
<?php
/*Create the array for example */
$data[0]['city'] = "LONDON";
$data[0]['country'] = "ENGLAND";
$data[1]['city'] = "LONDON";
$data[1]['country'] = "ENGLAND";
$data[2]['city'] = "LONDON";
$data[2]['country'] = "ENGLAND";
$data[3]['city'] = "PARIS";
$data[3]['country'] = "FRANCE";
$data[4]['city'] = "LIVERPOOL";
$data[4]['country'] = "ENGLAND";
$data[5]['city'] = "ROME";
$data[5]['country'] = "ITALY";
$data[6]['city'] = "ROME";
$data[6]['country'] = "ITALY";
$data[7]['city'] = "PARIS";
$data[7]['country'] = "FRANCE";
$data[8]['city'] = "BRISTOL";
$data[8]['country'] = "ENGLAND";
/* print the array for show
echo '<pre>';
print_r($data);*/
foreach($data as $val){
if(!isset($newData[$val['country']][$val['city']])){
$newData[$val['country']][$val['city']] = 1;
} else {
++$newData[$val['country']][$val['city']];
}
$sorted["{$val['country']} - {$val['city']}"] = $newData[$val['country']][$val['city']];
}
/* print created array */
echo '<pre>';
print_r($newData);
/* print the sorted array */
arsort($sorted);
print_r($sorted);
Will return:
Array
(
[ENGLAND] => Array
(
[LONDON] => 3
[LIVERPOOL] => 1
[BRISTOL] => 1
)
[FRANCE] => Array
(
[PARIS] => 2
)
[ITALY] => Array
(
[ROME] => 2
)
)
Array
(
[ENGLAND - LONDON] => 3
[FRANCE - PARIS] => 2
[ITALY - ROME] => 2
[ENGLAND - LIVERPOOL] => 1
[ENGLAND - BRISTOL] => 1
)
Edit - Added select from $newData:
Example of select - just to illustrate how to loop and get your values and keys:
?><select id="city" name="city"><?php
foreach($newData as $country => $cities){
foreach($cities as $city => $quantity){
?>
<option id="<?php echo $city;?>" value='<?php echo "$country-$city";?>' ><?php echo $city;?></option>
<?php
}
}
?></select><?php
Will return:
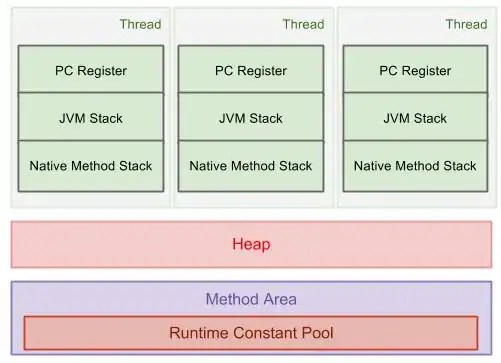
Which is:
<select id="city" name="city">
<option id="LONDON" value="ENGLAND-LONDON">LONDON</option>
<option id="LIVERPOOL" value="ENGLAND-LIVERPOOL">LIVERPOOL</option>
<option id="BRISTOL" value="ENGLAND-BRISTOL">BRISTOL</option>
<option id="PARIS" value="FRANCE-PARIS">PARIS</option>
<option id="ROME" value="ITALY-ROME">ROME</option>
</select>
Edit - if you want this: all data sorted and countries
Do this:
add
$sorted2[$val['city']] = $newData[$val['country']][$val['city']];
$countries[$val['city']] = $val['country'];
to the first foreach after $sorted...
after the loop add:
arsort($sorted2);
And in the select loop do this:
?><select id="city" name="city"><?php
foreach($sorted2 as $city => $quantity){
?>
<option id="<?php echo $city;?>" value='<?php echo $city;?>' ><?php echo "$city $countries[$city] ($quantity)";?></option>
<?php
}
?></select><?php