I ended up coding a tag editor myself since I didn't want to use a library. It's for ID3v1 tags only.
Full Code
Here's how it works:
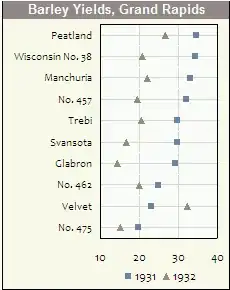
So all you have to do is write 128 bytes to the end of the file. Starting with TAG
, followed by 30 bytes for the title, 30 for the artist, etc. The genre list can be found here.
public void writeID3v1Tags(File file, String title, String artist, String album, String year, String comment, int genreId) { throws IOException {
RandomAccessFile randomAccessFile = new RandomAccessFile(file, "r");
FileChannel channel = randomAccessFile.getChannel();
if (!hasID3v1Tag(channel)) {
channel.position(channel.size());
writeTag(channel, "TAG", 3);
}
writeTag(channel, title, 30);
writeTag(channel, artist, 30);
writeTag(channel, album, 30);
writeTag(channel, year, 4);
writeTag(channel, comment, 30);
writeTag(channel, String.valueOf((char) genreId), 1);
channel.close();
}
private void writeTag(FileChannel channel, String tagValue, int maxLength) throws IOException {
int length = tagValue.length();
if (length > maxLength) {
tagValue = tagValue.substring(0, maxLength);
length = tagValue.length();
}
ByteBuffer byteBuffer = ByteBuffer.wrap(tagValue.getBytes());
channel.write(byteBuffer);
byteBuffer = ByteBuffer.allocate(maxLength - length);
channel.write(byteBuffer);
}
private boolean hasID3v1Tag(FileChannel channel) throws IOException {
channel.position(channel.size() - 128);
String prefix = readTag(channel, 3);
return "TAG".equals(prefix);
}
If you want more tags (like album cover, lyrics, and much more) you need to use ID3v2. It works basically the same but is a little bit more complex, more info under https://id3.org/id3v2.3.0