You could query WMI
as I prefer to use OS tools (should work with psutil
aswell, as mentioned by @BaguetteYeeter):
import os
import subprocess
import sys
print("Python interpreter: %s" % sys.executable)
parentShellName = None
# root process to look for parents until we find a process name
# which has not python in it's name
parentPid = os.getpid()
while 1:
# In case of ipython the second parent process is the Shell, so we are looping!
# Probably there should be a counter to finish the while loop in case no shell could be detected!
cmd = 'wmic process where "ProcessId=%s" get parentprocessid /format:list' % parentPid
proc = subprocess.Popen(cmd, stdout=subprocess.PIPE, stderr=subprocess.PIPE, shell=True)
out, err = proc.communicate()
key, parentPid = out.strip().decode('utf-8').split('=')
print("Parent ProcessId: %s" % parentPid)
cmd2 = 'wmic process where "ProcessId=%s" get name /format:list' % parentPid
proc = subprocess.Popen(cmd2, stdout=subprocess.PIPE, stderr=subprocess.PIPE, shell=True)
out, err = proc.communicate()
key, parentShellName = out.strip().decode('utf-8').split('=')
if 'python' not in parentShellName.lower():
break
print(parentShellName)
Out:
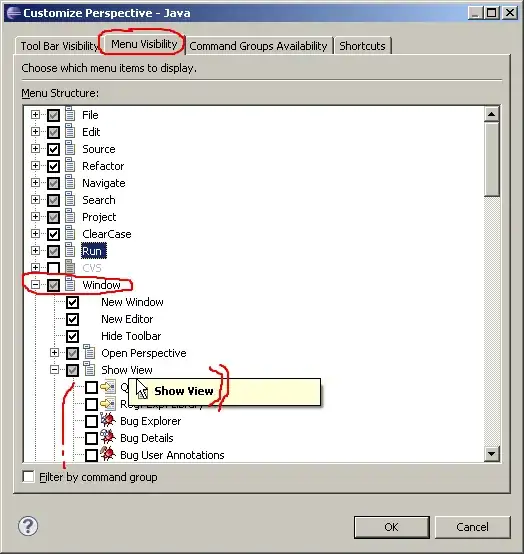