I would suggest extracting matching entries from the jar file and save to a temporary location to get the java.io.File reference.
Option #1:
If you are reading from a file system, use ZipFile to read the file then use ZipFile.getEntry("zip-path") to get the entry and save using Files.copy
See: ZipEntry to File
Option #2:
If you are reading from an input stream source, use ZipInputStream to read the jar file, then iterate, filter and apply action to matching entries. Each matching entry is coupled with a matching ZipInputStream and you can use those input streams to save them to a temporary location, then create the List<File> reference to hand off to another function.
I wrote a quick example in this repo:
The demo essentially just reads the jar file in the resource folder and finds a single matching zip entry (META-INF/license.txt) and saves it to a file.
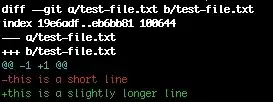
See Example Implementation in:
<script src="https://gist.github.com/nfet/27fce2870b8cd42e3337f6a21b8e9711.js"></script>