You can split text in multiple Run
s and use FontWeight
for your bold text.
<TextBlock>
<Run Text="Who is "/>
<Run FontWeight="Bold" Text="Tom"/>
<Run Text="? I need to find him!"/>
</TextBlock>
Or
<TextBlock>Who is <Bold>Tom</Bold>? I need to find him!</TextBlock>
Edited:
To be able to add different format in a single TextBlock
I think you maybe subscribe to TargetUpdated
event of your TextBlock
and add Run
class for each string between <b>
and </b>
Exemple for TargetUpdated
event:
private void MyText_TargetUpdated(object sender, DataTransferEventArgs e)
{
string text = myText.Text;
if (text.Contains("<b>") && text.Contains("</b>"))
{
int nrOfB = text.Split("<b>", StringSplitOptions.None).Length - 1;
myText.Text = "";
string textAfter ="";
for (int i = 0; i < nrOfB; i++)
{
int startIndex = text.IndexOf("<b>");
int endIndex = text.IndexOf("</b>");
string textBefore = text.Substring(0, startIndex);
string textBolded = text.Substring(startIndex + 3, endIndex - (startIndex + 3));
textAfter = text.Substring(endIndex + 4);
Debug.WriteLine($"Text Before: {textBefore},\nTextBolded: {textBolded},\nTextAfter: {textAfter}");
myText.Inlines.Add(new Run(textBefore));
myText.Inlines.Add(new Bold(new Run(textBolded)));
text = textAfter;
}
myText.Inlines.Add(new Run(textAfter));
}
}
and xaml control will be:
<UserControl.Resources>
<Style TargetType="local:Test">
<Setter Property="MyText" Value="{Binding MyTextVM, Mode=TwoWay}"/>
</Style>
</UserControl.Resources>
<TextBlock x:Name="myText" Text="{Binding ElementName=TestV, Path=MyText, Mode=OneWay, NotifyOnTargetUpdated=True}" TargetUpdated="MyText_TargetUpdated"/>
local:Test - my View,
TestV - Name for the view,
MyText - DependencyProperty of my custom TextBlock class,
MyTextVM - property from ViewModel
This string: My Text is a <b>Bold</b> text. <b>Second</b> text bold is <b>Bolded</b> too.
will create this visual effect:
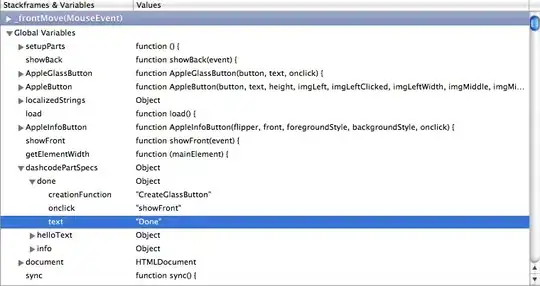