Depending on where you want these labels you have a couple options. If you want to set the labels along the bottom of the chart, you can use the IndexAxisValueFormatter(labels)
on the x axis. If you want to add labels on the top of the bars you can set a ValueFormatter
on the BarDataSet
. Here is an example that sets "L" or "M" on the lower axis depending on the bar height, and "Less" or "More" on the bar label itself - also depending on the bar height.
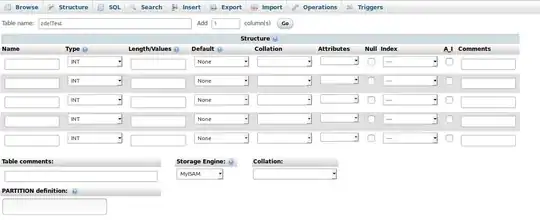
For setting the x axis labels, the relevant command is
xaxis.valueFormatter = IndexAxisValueFormatter(labels)
and for the bar labels
barDataSet.valueFormatter = object : ValueFormatter() {
override fun getBarLabel(barEntry: BarEntry?): String {
var label = ""
barEntry?.let { e ->
// here you can access both x and y
label = if( e.y > 25 ) {
"More"
} else {
"Less"
}
}
return label
}
}
If you want the labels above the bars, you can use
barChart.setDrawValueAboveBar(true)
Complete Example
val barChart = findViewById<BarChart>(R.id.bar_chart)
// the values on your bar chart - wherever they may come from
val yVals = listOf(10f, 20f, 30f, 40f, 50f, 60f)
val valueSet1 = ArrayList<BarEntry>()
val labels = ArrayList<String>()
// You can create your data sets and labels at the same time -
// then you have access to the x and y values and can
// determine what labels to set
for (i in yVals.indices) {
val yVal = yVals[i]
val entry = BarEntry(i.toFloat(), yVal)
valueSet1.add(entry)
if( yVal > 20) {
labels.add("M")
}
else {
labels.add("L")
}
}
val dataSets: MutableList<IBarDataSet> = ArrayList()
val barDataSet = BarDataSet(valueSet1, " ")
barChart.setDrawBarShadow(false)
barChart.setDrawValueAboveBar(false)
barChart.description.isEnabled = false
barChart.setDrawGridBackground(false)
val xaxis: XAxis = barChart.xAxis
xaxis.setDrawGridLines(false)
xaxis.position = XAxis.XAxisPosition.BOTTOM
xaxis.granularity = 1f
xaxis.isGranularityEnabled = true
xaxis.setDrawLabels(true)
xaxis.setDrawAxisLine(false)
xaxis.valueFormatter = IndexAxisValueFormatter(labels)
xaxis.textSize = 15f
val yAxisLeft: YAxis = barChart.axisLeft
yAxisLeft.setPosition(YAxis.YAxisLabelPosition.OUTSIDE_CHART)
yAxisLeft.setDrawGridLines(true)
yAxisLeft.setDrawAxisLine(true)
yAxisLeft.isEnabled = true
yAxisLeft.textSize = 15f
barChart.axisRight.isEnabled = false
barChart.extraBottomOffset = 10f
barChart.extraLeftOffset = 10f
val legend: Legend = barChart.legend
legend.isEnabled = false
barDataSet.color = Color.CYAN
barDataSet.valueTextSize = 15f
// You can also set a value formatter on the bar data set itself (and enable
// setDrawValues). In this you get a BarEntry which has both the x position
// and bar height.
barDataSet.setDrawValues(true)
barDataSet.valueFormatter = object : ValueFormatter() {
override fun getBarLabel(barEntry: BarEntry?): String {
var label = ""
barEntry?.let { e ->
// here you can access both x and y
// values with e.x and e.y
label = if( e.y > 25 ) {
"More"
} else {
"Less"
}
}
return label
}
}
dataSets.add(barDataSet)
val data = BarData(dataSets)
barChart.data = data
barChart.invalidate()