Detailed Answer for those who are also trapped in such issues.
Finally now I'm able to design the screen in a way I want,
According to my question it was becoming difficult for me to put view on
top of another view, due to a reason, which was this that my Root View (View A), was totally built using borders.
Question: Why I'm using just border?
Answer: Actually I wanted to design background of my screen in such way(diagonally intersected), which was easy for me to build in a triangular way,
It is also a detailed discussion how triangles can be created, although there were many ways, but I opt the one which was easy for me.
I don't want to go in detailed discussion about triangle formation, I have already answered it on stackoverflow interested people can check out:
Now I'm assuming nobody would have an issue in this matter that why I'm using just borders.
Coming Back to actual issue, I had just borders in main/root View (View A), means having no space inside the view to accomodate other Views as a Child.
I tried my things but was not working...
People are talking about some fancy words like zIndex, relative position, absolute position.
- zIndex: lets the developer control the order in which components are displayed over one another.
- relative position: it is default position of every component in each react native, which means our components will follow the normal order (in which components are written)
- absolute position: you can explicitly change position of any component to absolute and that component now will not follow the order it will move to the top position inside the parent component (or to the top any direction which is mentioned by developer using Flex Direction)
As I didn't had space inside View A in my case, so it is even not looking apparently fine to put something inside a view having no space (just borders).
So I made a new parent on top of View A and View B, and my issue is resolved, because View A and View B are locating at same level, so we don't care eithor View A has a space inside it or not, we can simply overlap both Views...
Before showing exact code let me give some examples, According to the code below, all the Views will be layed out one after other, and all have default position (relative)
const App = () => {
const Height=Dimensions.get("screen").height
const Width=Dimensions.get("screen").width
return <View style={{height:Height,width:Width}}>
<View style={{height:300,width:300,backgroundColor:'purple',}}></View>
<View style={{height:200,width:200,backgroundColor:'blue',}}></View>
<View style={{height:100,width:100,backgroundColor:'gray',}}></View>
</View>
}
But as i change position of all views to absolute, they all move to the top of their primaryAxis (primaryAxis is defined by flexDirection it could be row or colum, by default it is column in react-native)...
const App = () => {
const Height=Dimensions.get("screen").height
const Width=Dimensions.get("screen").width
return <View style={{height:Height,width:Width}}>
<View style=
{{height:300,width:300,backgroundColor:'purple',position:'absolute'}}></View>
<View style=
{{height:200,width:200,backgroundColor:'blue',position:'absolute'}}></View>
<View style=
{{height:100,width:100,backgroundColor:'gray',position:'absolute'}}></View>
</View>
}
I fix my code like this.
const App = () => {
const Height=Dimensions.get("screen").height
const Width=Dimensions.get("screen").width
return <View style={{height:Height,width:Width}}>
<View style={{
flex:1,
borderTopWidth:Height/2,
borderBottomWidth:Height/2,
borderRightWidth:Width/2,
borderLeftWidth:Width/2,
borderTopColor:"gray",
borderBottomColor:"red",
borderRightColor:"red",
borderLeftColor:"gray",
}}></View>
<View style={{
position:'absolute',
height:Height,
width:Width,
backgroundColor:'transparent',
justifyContent:'center',
alignItems:'center'
}}>
<View style=
{{height:100,width:100,backgroundColor:'purple',borderRadius:20,margin:10}}>
</View>
<View style=
{{height:100,width:100,backgroundColor:'cyan',borderRadius:20,margin:10}}>
</View>
<View style=
{{height:100,width:100,backgroundColor:'pink',borderRadius:20,margin:10}}>
</View>
</View>
</View>;
}
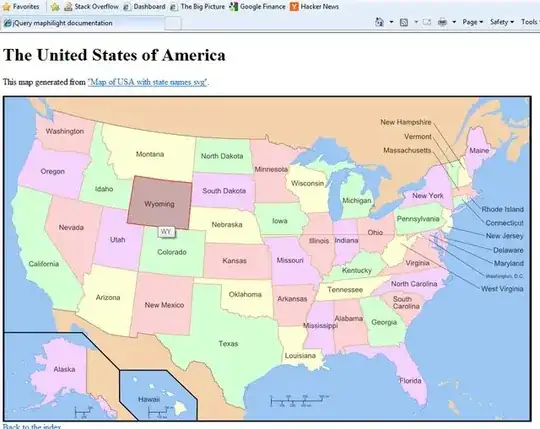