OK, so you're making you a settings form. Let's do a quick tut on how Microsoft intended stuff like this be done.
Rename your form SettingsForm. I've made a project with a new form, called SettingsForm, and I've added a combo, a numericupdown and a textbox. If you add these too, you can follow along with these steps
Look in the properties grid for the numeric updown. At the top is (Application Settings), expand it to see Property Binding. Click the 3 dots next to Property Binding, a new window opens probably pointing at Value. Drop down next to value and choose New (because you don't have any settings yet)
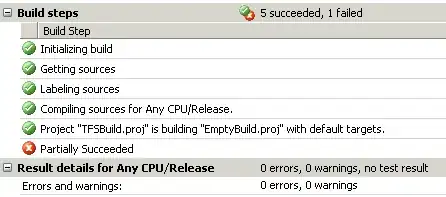
Write something like ComPort in here and choose a reasonable starting value, like 1. OK
Same for the Textbox. Here's one I made earlier:
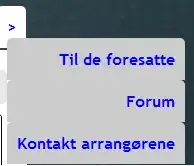
- For the combo, put some strings into its Items
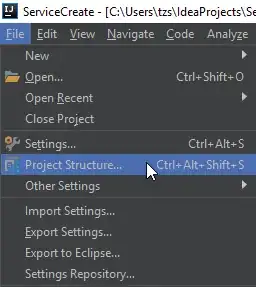
- And in the property settting, bind Text, and put a same entry as one of the items

- Pretty much that's all you have to do. The controls on this form will now edit the values of
Properties.Settings.Default.XXX
when the user changes them, where XXX is the names you chose. Here are the settings on the Project menu.. Project properties.. Settings tab
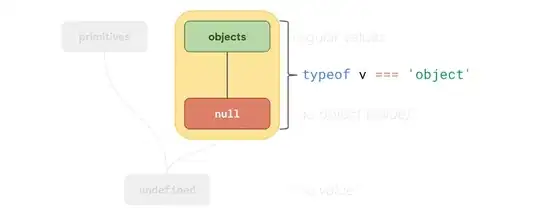
- Here's a lovely Main Form, very simple - two buttons, one shows a messagebox of whatever is selected for Baud, the other opens the settings window to select baud etc
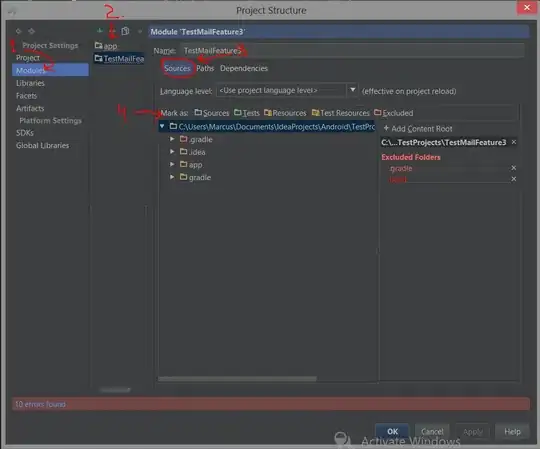
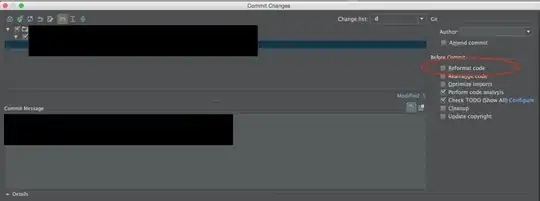
- it's even so magic,that you can open lots of SettingsForms, change one and they all change (not that you need this..) but it shows if you set a value from code, then the UI would change to reflect it too (two way binding)
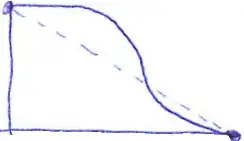
So.. All you have to do, after binding your controls properties to various settings, is read them into your com port e.g.:
var comPort = new SomeComportLibraryThing();
comPort.Port = "COM" + Properties.Settings.Default.Comport; //it's just a number from 1 to 9, add a string onto the start
comPort.Baud = int.Parse(Properties.Settings.Default.Baud);
comPort.Send(Properties.Settings.Default.ModemInitString);
Or however you need - these are just variables like anything else