Ensure that you have the correct VS Workloads and Individual Components installed.
- Open Visual Studio Installer
- Click Modify
- Click Workloads tab
- Ensure ASP.NET and web development is checked, if not, check it.
- Click Individual components
- Check desired .NET Framework SDK's and targeting pack (ie:
.NET Framework 4.7.2 SDK
, .NET Framework 4.7.2 targeting pack
, .NET Framework 4.8 SDK
, and .NET Framework 4.8 targeting pack
)
- If you made any changes, in lower-right corner, select Download all, then install. Then click Modify.
Then try the following:
Note: The code below is converted to VB.NET from here. However, the class name was changed. For testing, I used .NET Framework version 4.8, but other versions may work.
VS 2019:
Create a new project
Open Visual Studio
Click Continue without code
Click File
Select New
Select Project
Select the following:

Then, select:
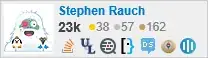
Click Next
Enter desired project name (ex: RSSFeedReader)
Click Create
Select the following:

Optional: On right-side, under Advanced, uncheck Configure for HTTPS
Click Create
Open Solution Explorer
- In VS menu, click View
- Select Solution Explorer
Add class (name: RSSFeed.vb)
- In Solution Explorer, right-click <project name> (ex: RSSFeedReader)
- Select Add
- Select Class... (name: RSSFeed.vb)
- Click Add
RSSFeed.vb
Public Class RSSFeed
Public Property Title As String
Public Property Link As String
Public Property PublishDate As String
Public Property Description As String
End Class
Add WebForm (name: default.aspx)
- In Solution Explorer, right-click <project name> (ex: RSSFeedReader)
- Select Add
- Select New Item...
- Select Web Form (name: default.aspx)
- Click Add
Modify default.aspx
- In Solution Explorer, right-click default.aspx
- Select View Markup
default.aspx:
<%@ Page Language="vb" AutoEventWireup="false" CodeBehind="default.aspx.vb" Inherits="RSSFeedReader._default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<h3>Read RSS Feeds</h3>
<form id="Form1" runat="server" >
<!-- Where XYZ refers to the publication from where you wish to fetch the RSS feed from -->
<div style="max-height:350px; overflow:auto">
<asp:GridView ID="gvRss" runat="server" AutoGenerateColumns="false" ShowHeader="false" Width="90%">
<Columns>
<asp:TemplateField>
<ItemTemplate>
<table width="100%" border="0" cellpadding="0" cellspacing="5">
<tr>
<td>
<h3 style="color:#3E7CFF"><%#Eval("Title") %></h3>
</td>
<td width="200px">
<%#Eval("PublishDate") %>
</td>
</tr>
<tr>
<td colspan="2">
<hr />
<%#Eval("Description") %>
</td>
</tr>
<tr>
<td> </td>
<td align="right">
<a href='<%#Eval("Link") %>' target="_blank">Read More...</a>
</td>
</tr>
</table>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
</div>
</form>
</body>
</html>
Modify default.aspx.vb
- In Solution Explorer, right-click default.aspx
- Select View Code
default.aspx.vb:
Public Class _default
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
'ToDo: replace with the URL to your desired RSS feed
Dim rssFeedUrls As List(Of String) = New List(Of String)()
'add desired URLs
rssFeedUrls.Add("https://www.nasa.gov/rss/dyn/lg_image_of_the_day.rss")
rssFeedUrls.Add("http://thehill.com/rss/syndicator/19110")
'get data
PopulateRssFeed(rssFeedUrls)
End Sub
Private Sub PopulateRssFeed(rssFeedUrls As List(Of String))
'create new List
Dim feeds As List(Of RSSFeed) = New List(Of RSSFeed)
Dim currentURL As String = String.Empty
Try
For Each url As String In rssFeedUrls
'set value
currentURL = url
'create new instance
Dim xDoc As XDocument = New XDocument()
'load
xDoc = XDocument.Load(url)
Dim items = From x In xDoc.Descendants("item")
Select New RSSFeed With
{
.Title = x.Element("title").Value,
.Link = x.Element("link").Value,
.PublishDate = x.Element("pubDate").Value,
.Description = x.Element("description").Value
}
If items IsNot Nothing Then
For Each i In items
Dim f As RSSFeed = New RSSFeed() With {
.Title = i.Title,
.Link = i.Link,
.PublishDate = i.PublishDate,
.Description = i.Description
}
'add
feeds.Add(f)
Next
End If
Next
gvRss.DataSource = feeds
gvRss.DataBind()
Catch ex As Exception
'ToDo: replace with desired code
Dim errMsg As String = String.Format("Error (PopulateRssFeed): {0} (url: {1})", ex.Message, currentURL)
Debug.WriteLine(errMsg)
Throw ex
End Try
End Sub
End Class
Ensure the following IIS features are installed/turned on: (Win 7)
Open Control Panel
Select View by: Small icons
Click Programs and Features
On left side click Turn Windows features on or off
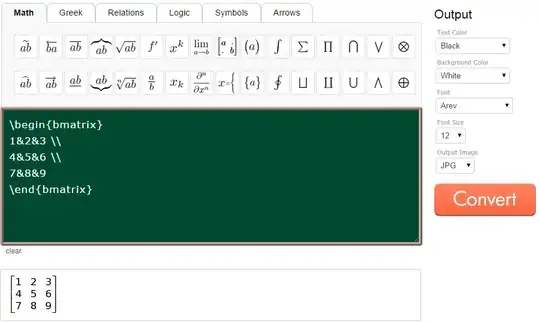
Expand Internet Information Services
Expand Web Management Tools
Check IIS Mangement Console

Expand World Wide Web Services
Expand Application Development Features
Check ASP.NET (checking this option will also check some other options)
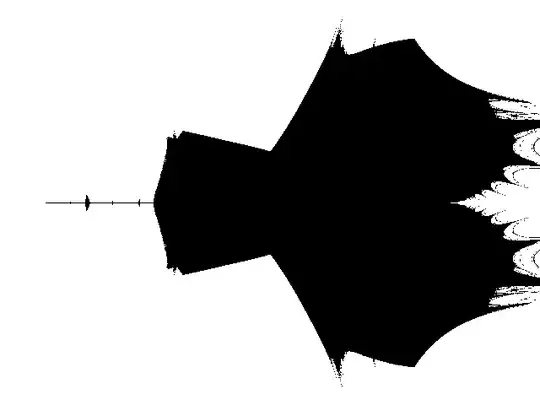
Click OK
Update:
_default
is defined in "default.aspx.designer.vb"
To open default.aspx.designer.vb:
- In Solution Explorer, right-click default.aspx
- Select View Code Gen File
default.aspx.designer.vb
'------------------------------------------------------------------------------
' <auto-generated>
' This code was generated by a tool.
'
' Changes to this file may cause incorrect behavior and will be lost if
' the code is regenerated.
' </auto-generated>
'------------------------------------------------------------------------------
Option Strict On
Option Explicit On
Partial Public Class _default
'''<summary>
'''Form1 control.
'''</summary>
'''<remarks>
'''Auto-generated field.
'''To modify move field declaration from designer file to code-behind file.
'''</remarks>
Protected WithEvents Form1 As Global.System.Web.UI.HtmlControls.HtmlForm
'''<summary>
'''gvRss control.
'''</summary>
'''<remarks>
'''Auto-generated field.
'''To modify move field declaration from designer file to code-behind file.
'''</remarks>
Protected WithEvents gvRss As Global.System.Web.UI.WebControls.GridView
End Class
Update 2:
When creating the project, for "Create a new ASP.NET Web Application" if you choose:

then use the following code in "Default.aspx" instead of the code above:
Default.aspx:
<%@ Page Title="Home Page" Language="VB" MasterPageFile="~/Site.Master" AutoEventWireup="true" CodeBehind="Default.aspx.vb" Inherits="RSSFeedReader._Default" %>
<asp:Content ID="BodyContent" ContentPlaceHolderID="MainContent" runat="server">
<h3>Read RSS Feeds</h3>
<!-- Where XYZ refers to the publication from where you wish to fetch the RSS feed from -->
<div style="max-height:350px; overflow:auto">
<asp:GridView ID="gvRss" runat="server" AutoGenerateColumns="false" ShowHeader="false" Width="90%">
<Columns>
<asp:TemplateField>
<ItemTemplate>
<table width="100%" border="0" cellpadding="0" cellspacing="5">
<tr>
<td>
<h3 style="color:#3E7CFF"><%#Eval("Title") %></h3>
</td>
<td width="200px">
<%#Eval("PublishDate") %>
</td>
</tr>
<tr>
<td colspan="2">
<hr />
<%#Eval("Description") %>
</td>
</tr>
<tr>
<td> </td>
<td align="right">
<a href='<%#Eval("Link") %>' target="_blank">Read More...</a>
</td>
</tr>
</table>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
</div>
</asp:Content>
Note: The code for "Default.aspx.vb" is the same.
Update 3:
According to this video, if you want to view a YT RSS feed, you need to use the following for the URL: https://www.youtube.com/feeds/videos.xml?channel_id=<channel_id>
Note: The <channel_id> is the last portion of the URL (ie: after the last /
). According to the video, there is a limit of 15 entries.
Also, one needs to use namespaces for the XML. Therefore, use the following for "default.aspx.vb":
default.aspx.vb:
Public Class _default
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
'ToDo: replace with the URL to your desired RSS feed
Dim rssFeedUrls As List(Of String) = New List(Of String)()
'add desired URLs
rssFeedUrls.Add("https://www.youtube.com/feeds/videos.xml?channel_id=UCBB7sYb14uBtk8UqSQYc9-w")
'get data
PopulateRssFeedYT(rssFeedUrls)
End Sub
Private Sub PopulateRssFeedYT(rssFeedUrls As List(Of String))
'create new List
Dim feeds As List(Of RSSFeed) = New List(Of RSSFeed)
Dim currentURL As String = String.Empty
Try
For Each url As String In rssFeedUrls
'set value
currentURL = url
'create new instance
Dim xDoc As XDocument = New XDocument()
'load
xDoc = XDocument.Load(url)
Dim items = From x In xDoc.Descendants("{http://www.w3.org/2005/Atom}entry")
Select New RSSFeed With
{
.Title = x.Element("{http://www.w3.org/2005/Atom}title").Value,
.Link = x.Element("{http://www.w3.org/2005/Atom}link").Attribute("href").Value,
.PublishDate = x.Element("{http://www.w3.org/2005/Atom}published").Value,
.Description = x.Element("{http://search.yahoo.com/mrss/}group").Element("{http://search.yahoo.com/mrss/}description").Value
}
If items IsNot Nothing Then
For Each i In items
Dim f As RSSFeed = New RSSFeed() With {
.Title = i.Title,
.Link = i.Link,
.PublishDate = i.PublishDate
}
If i.Description.Length <= 50 Then
f.Description = i.Description
Else
'only show the first 50 chars
f.Description = i.Description.Substring(0, 50)
End If
'add
feeds.Add(f)
Next
End If
Next
gvRss.DataSource = feeds
gvRss.DataBind()
Catch ex As Exception
'ToDo: replace with desired code
Dim errMsg As String = String.Format("Error (PopulateRssFeed): {0} (url: {1})", ex.Message, currentURL)
Debug.WriteLine(errMsg)
Throw ex
End Try
End Sub
End Class
Note: For alternative methods of retrieving the desired data the following may be helpful:
Resources: