I wrote bunch of codes which expands my accordion but here is a problem. When I click on button to expand accordion body, my listener calls twice.
Did anyone face this problem?
const accordionToggler = document.querySelectorAll('.accordion-toggler');
// expanding accordion by clicking on a single accordion toggler
accordionToggler.forEach(toggler => {
toggler.addEventListener('click', (e) => {
const caret = toggler.querySelector('.chevron-caret'); // chevron ico
if (caret.classList.contains('rotate')) {
caret.classList.remove('rotate')
} else {
caret.classList.add('rotate')
}
if (e.currentTarget.nextElementSibling.style.maxHeight === '' || e.currentTarget.nextElementSibling.style.maxHeight === '0px') {
e.currentTarget.nextElementSibling.style.maxHeight = toggler.nextElementSibling.scrollHeight + 'px';
} else {
e.currentTarget.nextElementSibling.style.maxHeight = 0;
}
console.log('menu loop function click');
});
console.log('menu loop function');
});
// expanding accordion by clicking on a single accordion toggler
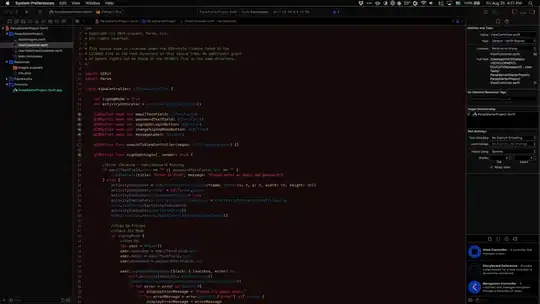