I believe there are a few ways you can achieve this Ahmet, I will go over one idea.
The interesting challenge from your question is that you don't only want to left align the image.
You want to:
- Left align the image with respect to the button title only
- However, the image along with the text should be center aligned as a whole within the UIButton
I created the below extension which gets you close to your desired result with some comments to explain my thought process:
extension UIButton
{
func configureWithLeftImage(_ imageName: String)
{
// Retrieve the desired image and set it as the button's image
let buttonImage = UIImage(named: imageName)?.withRenderingMode(.alwaysOriginal)
// Resize the image to the appropriate size if required
let resizedButtonImage = resizeImage(buttonImage)
setImage(resizedButtonImage, for: .normal)
// Set the content mode
contentMode = .scaleAspectFit
// Align the content inside the UIButton to be left so that
// image can be left and the text can be besides that on it's right
contentHorizontalAlignment = .left
// Set or compute the width of the UIImageView within the UIButton
let imageWidth: CGFloat = imageView!.frame.width
// Specify the padding you want between UIButton and the text
let contentPadding: CGFloat = 10.0
// Get the width required for your text in the button
let titleFrame = titleLabel!.intrinsicContentSize.width
// Keep a hold of the button width to make calculations easier
let buttonWidth = frame.width
// The UIImage and the Text combined should be centered so we need to calculate
// the x position of the image first.
let imageXPos = (buttonWidth - (imageWidth + contentPadding + titleFrame)) / 2
// Adjust the content to be centered
contentEdgeInsets = UIEdgeInsets(top: 0.0,
left: imageXPos,
bottom: 0.0,
right: 0.0)
}
// Make sure the image is sized properly, I have just given 50 x 50 as random
// Code taken from: https://www.createwithswift.com/uiimage-resize-resizing-an-uiimage/
private func resizeImage(_ image: UIImage?,
toSize size: CGSize = CGSize(width: 50, height: 50)) -> UIImage?
{
if let image = image
{
UIGraphicsBeginImageContextWithOptions(size, false, 0.0)
image.draw(in: CGRect(origin: CGPoint.zero, size: size))
let resizedImage = UIGraphicsGetImageFromCurrentImageContext()!
UIGraphicsEndImageContext()
return resizedImage
}
return nil
}
}
Then when you want to use it:
// Initialize a UIButton and set its frame
let customButton: UIButton = UIButton(type: .custom)
customButton.frame = CGRect(x: 100, y: 200, width: 150, height: 40)
// Customize the button as you wish
customButton.backgroundColor = .white
customButton.layer.cornerRadius = 5.0
customButton.setTitleColor(.black, for: .normal)
customButton.setTitle("смотрите", for: .normal)
// Call the function we just created to configure the button
customButton.configureWithLeftImage("play_icon")
// Finally add the button to your view
view.addSubview(customButton)
This is the end result produced which I believe is close to your desired goal:

This is the image I used as well inside the UIButton if you want to test it out
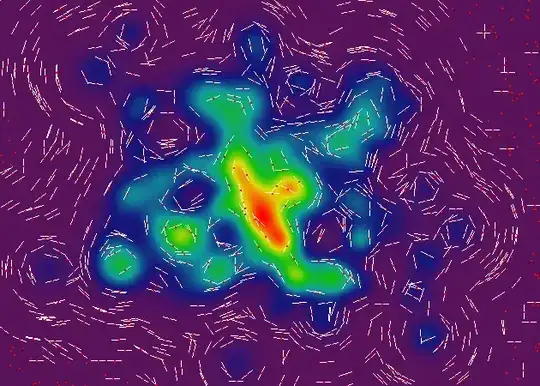
Update
The issue with your scenario was correctly identified by you in the comments, the size of your image was too large.
I have updated the above UIButton extension to include an image resize function which should solve the issue.
I have given random sizing for the UIImage inside the button, however you need to size it appropriate to your situation or dynamically calculate size of the image based on available space based on button size.
I also added some code for title insets to make final result better.
Now result works in PlayGround and iOS app:
App using your image
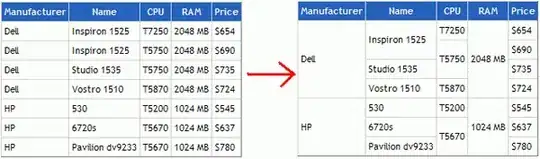
Playground using your image

Update 2.0
As Ahmet pointed out, there were issues calculating the right positions for the image and the title when the length of the string changes using titleInsets and imageInsets, sometimes leading to overlapping of the button and image.
Instead, Adjust the content inset instead as follows (updated in the extension above as well)
// The UIImage and the Text combined should be centered so we need to calculate
// the x position of the image first.
let imageXPos = (buttonWidth - (imageWidth + contentPadding + titleFrame)) / 2
// Adjust the content to be centered
contentEdgeInsets = UIEdgeInsets(top: 0.0,
left: imageXPos,
bottom: 0.0,
right: 0.0)
This will work if the text length of the button title is 1 or several characters