What's going wrong...
if (x < width) {
x = +10;
} else if (x >= 0) {
x = -10;
}
So, the above code is simply assigning either -10
or +10
to the x
variable, it never increments/decrements the value. Use +=
and -=
instead
if (x < width) {
x += 10;
} else if (x >= 0) {
x -= 10;
}
This will solve the immediate issue, but create a new one. It would be better to have a simple delta which was either positive or negative and is then simply applied to the variable
x += delta; // +/- speed
if (x + 20 >= width) {
x = width - 20;
delta *= -1
} ...
Additional fixes
Swing is not thread, you should not be using threads to change the state of the UI. Start by having a look at Concurrency in Swing and How to Use Swing Timers for more details.
You should also have a look at Painting in AWT and Swing and Performing Custom Painting as you should be preferring paintComponent
over paint
The following is a rewrite of your example using a Swing Timer
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ButtonGroup;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JToggleButton;
import javax.swing.Timer;
public class Test {
public static void main(String[] args) {
new Test();
}
public Test() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
JFrame frame = new JFrame();
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
protected class TestPane extends JPanel {
private AnimationPanel animationPane;
public TestPane() {
setLayout(new BorderLayout());
animationPane = new AnimationPanel();
add(animationPane);
JToggleButton pauseButton = new JToggleButton("Pause");
pauseButton.setSelected(true);
pauseButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
animationPane.setPaused(pauseButton.isSelected());
}
});
JButton fasterButton = new JButton("Faster");
fasterButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
animationPane.setSpeed(animationPane.getSpeed() * 2);
}
});
JButton slowerButton = new JButton("Slower");
slowerButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
animationPane.setSpeed(animationPane.getSpeed() / 2);
}
});
JToggleButton horizontalButton = new JToggleButton("Horizontal");
JToggleButton verticalButton = new JToggleButton("Vertical");
horizontalButton.setSelected(true);
ButtonGroup bg = new ButtonGroup();
bg.add(horizontalButton);
bg.add(verticalButton);
horizontalButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
animationPane.setLeftToRight(horizontalButton.isSelected());
}
});
verticalButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
animationPane.setLeftToRight(!verticalButton.isSelected());
}
});
JPanel actionPane = new JPanel();
actionPane.add(pauseButton);
actionPane.add(slowerButton);
actionPane.add(fasterButton);
actionPane.add(horizontalButton);
actionPane.add(verticalButton);
add(actionPane, BorderLayout.SOUTH);
}
}
public class AnimationPanel extends JPanel {
public int getSpeed() {
return speed;
}
public void setSpeed(int speed) {
this.speed = speed;
}
public boolean isLeftToRight() {
return isLeftToRight;
}
public void setLeftToRight(boolean isLeftToRight) {
this.isLeftToRight = isLeftToRight;
}
private boolean paused = true;
private int speed = 10;
private boolean isLeftToRight = true;
private Point origin = new Point(190, 190);
private Timer timer;
public AnimationPanel() {
timer = new Timer(16, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
movement();
repaint();
}
});
}
@Override
public Dimension getPreferredSize() {
return new Dimension(400, 400);
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g.create();
g2d.setColor(Color.BLUE);
g2d.fillOval(origin.x, origin.y, 20, 20);
g2d.dispose();
}
public boolean isPaused() {
return paused;
}
public void setPaused(boolean paused) {
this.paused = paused;
if (paused) {
timer.stop();
} else {
timer.start();
}
}
public void movement() {
int height = this.getHeight();
int width = this.getWidth();
if (isLeftToRight) {
origin.x += speed;
if (origin.x + 20 >= width) {
speed *= -1;
origin.x = width - 20;
} else if (origin.x <= 0) {
speed *= -1;
origin.x = 0;
}
} else {
origin.y += speed;
if (origin.y + 20 >= height) {
speed *= -1;
origin.y = height - 20;
} else if (origin.y <= 0) {
speed *= -1;
origin.y = 0;
}
}
}
}
}
A "different" approach
I don't like delta based animations, I think they are shorted sighted and they generally produce bad results. Where possible, I prefer to make use of time based animations. That is, based on a given time, move the object over a given range.
When done right, this is really flexible. It allows the system to drop frames automatically without the animation "stalling" and generally produces nicer animation which is generally easier to manage and maintained.
This does, however, introduce more complexity, but if you spend the time to generalise the workflows, they can be re-used relatively easily.
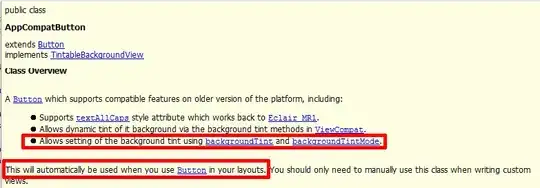
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.geom.Ellipse2D;
import java.awt.geom.Line2D;
import java.awt.geom.Point2D;
import java.time.Duration;
import java.time.Instant;
import javax.swing.ButtonGroup;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JToggleButton;
import javax.swing.Timer;
public class Test {
public static void main(String[] args) {
new Test();
}
public Test() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
JFrame frame = new JFrame();
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
protected class TestPane extends JPanel {
private AnimationPanel animationPane;
public TestPane() {
setLayout(new BorderLayout());
animationPane = new AnimationPanel();
add(animationPane);
JToggleButton pauseButton = new JToggleButton("Run");
pauseButton.setSelected(true);
pauseButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (pauseButton.isSelected()) {
pauseButton.setText("Run");
} else {
pauseButton.setText("Pause");
}
animationPane.setPaused(pauseButton.isSelected());
}
});
// JButton fasterButton = new JButton("Faster");
// fasterButton.addActionListener(new ActionListener() {
// @Override
// public void actionPerformed(ActionEvent e) {
// animationPane.setSpeed(animationPane.getSpeed() * 2);
// }
// });
// JButton slowerButton = new JButton("Slower");
// slowerButton.addActionListener(new ActionListener() {
// @Override
// public void actionPerformed(ActionEvent e) {
// animationPane.setSpeed(animationPane.getSpeed() / 2);
// }
// });
JToggleButton horizontalButton = new JToggleButton("Horizontal");
JToggleButton verticalButton = new JToggleButton("Vertical");
horizontalButton.setSelected(true);
ButtonGroup bg = new ButtonGroup();
bg.add(horizontalButton);
bg.add(verticalButton);
// horizontalButton.addActionListener(new ActionListener() {
// @Override
// public void actionPerformed(ActionEvent e) {
// animationPane.setLeftToRight(horizontalButton.isSelected());
// }
// });
//
// verticalButton.addActionListener(new ActionListener() {
// @Override
// public void actionPerformed(ActionEvent e) {
// animationPane.setLeftToRight(!verticalButton.isSelected());
// }
// });
JPanel actionPane = new JPanel();
actionPane.add(pauseButton);
// actionPane.add(slowerButton);
// actionPane.add(fasterButton);
// actionPane.add(horizontalButton);
// actionPane.add(verticalButton);
add(actionPane, BorderLayout.SOUTH);
}
}
public class AnimationPanel extends JPanel {
public enum Direction {
VERTICAL, HORIZONTAL
}
private Direction direction = Direction.HORIZONTAL;
private Point2D origin = new Point2D.Double(200, 200);
private Animator animator;
private Range<Double> range;
private Duration duration = Duration.ofSeconds(5);
private Ellipse2D dot = new Ellipse2D.Double(0, 0, 20, 20);
public AnimationPanel() {
animator = new Animator(new Animator.Observer() {
@Override
public void animatorDidTick(Animator animator, double progress) {
double nextValue = range.valueAt(progress);
if (direction == Direction.HORIZONTAL) {
origin.setLocation(nextValue, origin.getY());
}
repaint();
}
@Override
public void animatorDidComplete(Animator animator) {
double targetPoint = range.getTo();
if (direction == Direction.HORIZONTAL) {
range = getDotHorizontalRange();
if (targetPoint != range.getFrom()) {
range.reverse();
}
}
animator.setDuration(duration);
resume();
}
});
}
@Override
public Dimension getPreferredSize() {
return new Dimension(400, 400);
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g.create();
g2d.setColor(Color.BLUE);
g2d.drawRect(0, 0, getWidth() - 1, getHeight() - 1);
g2d.draw(new Line2D.Double(0, getHeight() / 2, getWidth(), getHeight() / 2));
g2d.draw(new Line2D.Double(getWidth() / 2, 0, getWidth() / 2, getHeight()));
g2d.translate(origin.getX() - (dot.getWidth() / 2d), origin.getY() - (dot.getHeight() / 2d));
g2d.fill(dot);
g2d.dispose();
}
protected Range<Double> getDotHorizontalRange() {
return new DoubleRange(dot.getWidth() / 2, getWidth() - (dot.getWidth() / 2));
}
protected double getHorizontalRangeDistance() {
return ((DoubleRange)getDotHorizontalRange()).getDistance();
}
public void setPaused(boolean paused) {
if (paused) {
animator.pause();
} else {
if (range == null) {
initialiseRange();
}
animator.resume();
}
}
protected void resume() {
if (range == null) {
// Try and force a restart...
setPaused(false);
}
animator.resume();
}
protected void initialiseRange() {
if (direction == Direction.HORIZONTAL) {
double currentX = origin.getX();
// Assume a positive intial direction
double avaliableRange = Math.abs(getHorizontalRangeDistance());
double distance = avaliableRange - currentX;
int remainingTime = (int)(duration.toMillis() * (distance / avaliableRange));
animator.setDuration(Duration.ofMillis(remainingTime));
range = new DoubleRange((double)currentX, getDotHorizontalRange().getTo());
}
}
}
public abstract class Range<T> {
private T from;
private T to;
public Range(T from, T to) {
this.from = from;
this.to = to;
}
public T getFrom() {
return from;
}
public T getTo() {
return to;
}
@Override
public String toString() {
return "From " + getFrom() + " to " + getTo();
}
public abstract T valueAt(double progress);
public void reverse() {
T nextFrom = to;
to = from;
from = nextFrom;
}
}
public class DoubleRange extends Range<Double> {
public DoubleRange(Double from, Double to) {
super(from, to);
}
public Double getDistance() {
return getTo() - getFrom();
}
@Override
public Double valueAt(double progress) {
double distance = getDistance();
double value = distance * progress;
value += getFrom();
return value;
}
}
public class Animator {
public enum State {
STOP, PAUSE, RUN
}
public interface Observer {
public void animatorDidTick(Animator animator, double progress);
public void animatorDidComplete(Animator animator);
}
private Duration duration = Duration.ofSeconds(5);
// Used to manage pause support. This will be
// added onto the "live" runtime when the
// animator is running
private Duration previousRuntime = Duration.ZERO;
private Instant epoch;
private Observer observer;
private State state = State.STOP;
// This is actually used to manage the "ticks"
private Timer ticker = new Timer(5, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (epoch == null) {
epoch = Instant.now();
}
double progress = getProgressAtCurrentTime();
observer.animatorDidTick(Animator.this, Math.max(0, Math.min(1.0, progress)));
if (progress >= 1.0) {
progress = 1.0;
stop();
observer.animatorDidComplete(Animator.this);
}
}
});
public Animator(Observer observer) {
this.observer = observer;
}
public void setDuration(Duration duration) {
this.duration = duration;
}
public boolean isPaused() {
return state == State.PAUSE;
}
public boolean isRunning() {
return state == State.RUN;
}
public boolean isStopped() {
return state == State.STOP;
}
public void pause() {
ticker.stop();
if (epoch != null) {
Duration runtime = Duration.between(epoch, Instant.now());
previousRuntime = previousRuntime.plus(runtime);
state = State.PAUSE;
}
epoch = null;
}
public void resume() {
state = State.RUN;
ticker.start();
}
protected double getProgressAtCurrentTime() {
Duration runtime = Duration.ZERO;
if (epoch != null) {
// The delta time between when we started and now
runtime = Duration.between(epoch, Instant.now());
}
// Plus any additonal time which was recored
runtime = runtime.plus(previousRuntime);
return runtime.toMillis() / (double) duration.toMillis();
}
// This is for internal reset purposes
protected void stop() {
ticker.stop();
state = State.STOP;
previousRuntime = Duration.ZERO;
epoch = null;
}
}
}
The above example makes use of a concept of "normalised" time. That is, any given animation transitions over a time range of 0-1. This makes it incredibly easy to change the speed. Want to to go faster? Decrease the duration. Slower? Increase the duration. Everything else is done by simply calculating the required properties against a "from" and "to" state and the current "normalised time" value.
For example, look at the animation above. The dot starts at the halfway point, but the time it takes to get to the other side is no different then the time it takes to return ALL the way to far side (the speed doesn't change), this is because, the initial duration is calculated based on a delta of the whole range and the current position (that is, the initial duration is 50% of the desired duration).
The above example also allows you to "pause" the running animation and when it resumes, it will continue as if nothing happened.
Animation, I mean, really good animation, is complicated. Don't believe me, take a look at move dot between 2 points jframe (which I've spent the last few days building out as personal projects to run in Java and MacOS, because apparently I don't have a live) and How can I implement easing functions with a thread which takes a deep dive into just one aspect of the animation theory and don't even get me started on "time line" animation.