By far the easiest solution in this case it to just use the inbuilt Dynamic Layout functionality:
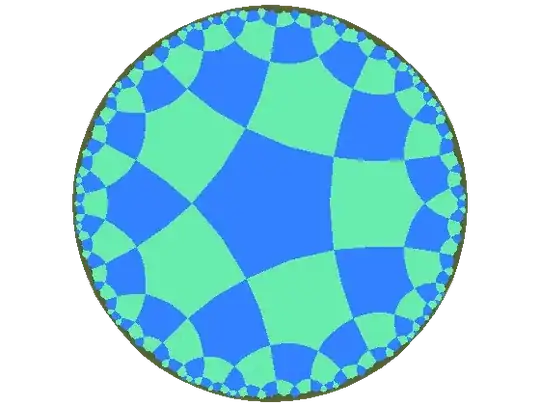
- Select the control in the IDE.
- Set the Sizing Type property to Both.
- Set Sizing X and Sizing Y to 100.
Then, as long as your dialog Border property is set to Resizing the tree control will stretch as you wanted:

No coding required as you can see:
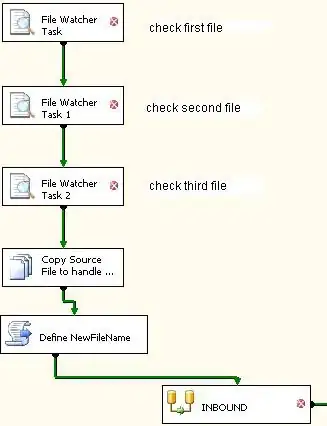
Much easier!
Manual Method
But, if you do things manually you need to be sure that you understand what type of coordinates the API calls provide you. Coordinates can be relative:
- Screen Coordinates
- Client Coordinates
For example:
The dimensions are given in screen coordinates relative to the upper-left corner of the display screen. The dimensions of the caption, border, and scroll bars, if present, are included.
The client coordinates specify the upper-left and lower-right corners of the client area. Since client coordinates are relative to the upper-left corners of the CWnd client area, the coordinates of the upper-left corner are (0,0).
For a top-level CWnd
object, the x and y parameters are relative to the upper-left corner of the screen. For a child CWnd
object, they are relative to the upper-left corner of the parent window's client area.
Your tree control is a child so when you move it, the coordinates need to be relative to the upper-left corner of the parent window's client area.
Note, there is a handy CWnd::ScreenToClient
API to translate from one system to the other. But this is not required in this instance.
Manual Method — Example
Putting it all together:
CRect rct;
GetClientRect(&rct); // The client area of your window (in client coordinates)
rct.top += N; // Adjust the top margin as required
myTree.MoveWindow(&rct); // Now move the child control
Please note that I have not debugged this to verify the code but it conveys the ideas.
Where possible I use the built in dynamic resizing, althought it can be very tricky to work out the sizing values. But in your case it is simple - 100.
I should point out that the above code will manually get it to the right place. But then you have to resize it as the window resizes. Try the Dynamic Layout approach instead.