Here's a simpler replication of the issue:
const obj = {
XMl: 1,
SFJ: 1,
dTg: 1,
amN: 1,
};
console.log(obj);
For which I get:
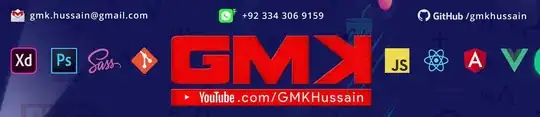
The summary seems to be shown in property order (more on that in a moment), but then when displaying the object details Chrome's devtools shows the properties in alphabetical order (including grouping capitals together; it looks very much like the ordering simple <
and >
would do, rather than localeCompare
or similar). Firefox's looks similar.
The first is covered by the JavaScript specification (details here). I don't think there's any standard that covers the second, but if you think about it, alpha order is probably more useful than property order when you're trying to find a property in an object with lots of properties. Property order is complicated and only mostly based on the order in which the properties were created, so it can can seem chaotic. And grouping the capitals together categorizes slightly, given the JavaScript convention that initial capital letters are almost entirely reserved for constructor functions and all caps constants.
Originally you used the term "associative array" instead of object. As I mentioned in a comment, they're really objects, not just associative arrays. If you did want just a basic "map" style name/value pairs thing, that would be a Map
and the order of map entries is nice and simple: It's the order the entry was created. If we do the same thing we did above but with a Map
, both the summary and the detail show the information in the same order:
const map = new Map([
["XMl", 1],
["SFJ", 1],
["dTg", 1],
["amN", 1],
]);
console.log(map);
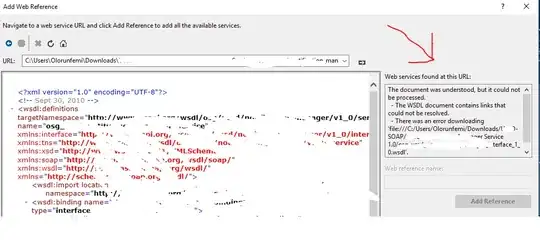