Why is it true
?
Java evaluates your code in a different order than you might expect. When you write
System.out.println("Result is: " + x instanceof Object);
what Java executes is:
- String concatenation
"Result is: " + x
resulting in the string "Result is: null
, then
- checking that string against
instanceof Object
, so "Result is: null" instanceof Object
which is obviously true
.
I.e. it executes it as if you would put paranthesis like this:
System.out.println(("Result is: " + x) instanceof Object);
This is due to the operator precedence rules in Java, which you can read more about here: Operators
You can see that addition +
binds stronger than instanceof
:
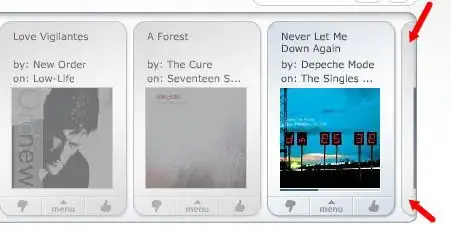
How to get the expected output?
In order to get the expected output, you have to make the order explicit by using paranthesis as follows:
System.out.println("Result is: " + (x instanceof Object));
// Result is: false
alternatively, split the expression into multiple by using some variables:
boolean isObject = x instanceof Object;
System.out.println("Result is: " + isObject);
Arguably also more readable.
As a general note, never rely on operator precedence rules (unless maybe *
and +
in a math context), otherwise you will only confuse readers.