There is a lot more going on in your math than you assume! You have three different primitive types there:
- 100.1 is a Double
- 100 is an Integer
- diff is a Decimal
If you put Option Strict On
at the top of your code, your code will not compile. This will tell you
Option Strict On disallows implicit conversions from 'Double' to 'Decimal'.
So you must either explicitly cast (this is what your Option Strict Off
version is doing implicitly)
Dim diff As Decimal = CDec(100.1 - 100)
but since it's the same thing you are already doing implicitly, you will get that same floating point issue. The better thing to do is to start with a Decimal instead of Double in the first place, such as
Dim diff = 100.1D - 100
By using the Decimal literal D
at the end of the floating point number it actually does Decimal math, and the result:
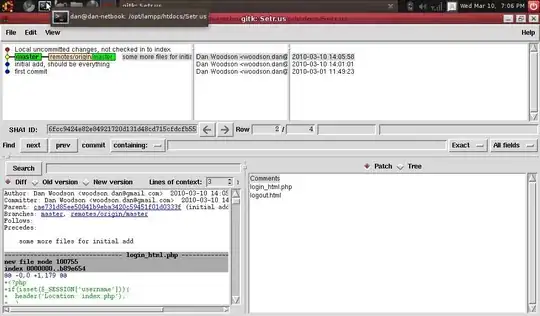