In a comment you've said:
I think now there are 3 items - Array.prototype
, Array.__proto__
, and [[prototype]]
. I thought all 3 were just the same.
([[Prototype]]
is usually capitalized.)
That's the fundamental misunderstanding (that and looking at the wrong part of the console output; more below). They aren't. Here's what they are:
[[Prototype]]
is the name of the internal field of an object that says what that object's prototype is.
__proto__
is an accessor property defined by Object.prototype
that exposes the [[Prototype]]
internal field. As I've told you before, it's deprecated. Use Object.getPrototypeOf
and (if you can't avoid it) Object.setPrototypeOf
.
SomeConstructorFunction.prototype
is a property on SomeConstructorFunction
that refers to an object. If you create an object via new SomeConstructorFunction
(or an equivalent operation), the object that SomeConstructorFunction.prototype
refers to is set as the [[Prototype]]
of the new object.
See also the answers to this question.
In Browser there are some Global-Objects pre-built (Objects, String, Number, Date, etc). Mother of all such global-objects is "Object". As, this Object is the prototype of nearly all of the data-types present in JS (except only 2 - null and undefined).
No, Object
is a function. Object.prototype
is the mother of all objects — except the ones it isn't, because it's possible for an object not to inherit from it (or indeed, from anything). Object.create(null)
creates an object with no prototype object.
Can someone differentiate to me, what exactly is the difference between __proto__
and [[prototype]]
and why the values inside both are completely different?
They aren't (but keep reading). In the picture you've shown, you're looking at [[Prototype]]
on a function, the setter of the __proto__
accessor property. You're not looking at [[Prototype]]
on the same thing you're looking at __proto__
on.
In your picture you're basically looking at Object.prototype
(because you're looking at __proto__
on the result of new Object
). Chrome's devtools don't show the [[Prototype]]
internal field of Object.prototype
(surprisingly), but it they did it would be null
per specification.
Let's instead look at a simple object:
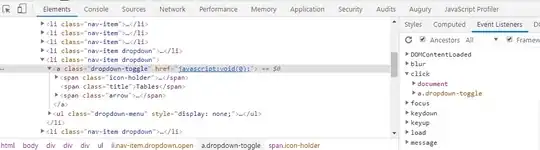
> const obj1 = {example: "x"};
<- undefined
> obj1
<- {example: 'x'}
example: "x"
[[Prototype]]: Object
> Object.getPrototypeOf(obj1) === Object.prototype
<- true
> Object.getPrototypeOf(obj1) === obj1.__proto__
<- true
> obj1.prototype
<- undefined
(One part of that which could be confusing is where is says [[Prototype]]: Object
. The devtools show the name of the constructor for an object. The [[Prototype]]
of the object is the object referenced by Object.prototype
, not the Object
constructor itself.)