Firstly :
Do not include Reality Composer's .rcproject
files in your archive for distribution. .rcproject
bundles contain the code with iOS 13.0+ classes, structs and enums. Instead, supply your project with USDZ files.
Secondly :
To allow iOS 13+ users to use RealityKit features, but still allow non-AR users to run this app starting from iOS 10.0, use the following code (CONSIDER, IT'S A SIMULATOR VERSION):
import UIKit
#if canImport(RealityKit) && TARGET_OS_SIMULATOR
import RealityKit
@available(iOS 13.0, *)
class ViewController: UIViewController {
var arView = ARView(frame: .zero)
override func viewDidLoad() {
super.viewDidLoad()
arView.frame = self.view.frame
self.view.addSubview(arView)
let entity = ModelEntity(mesh: .generateBox(size: 0.1))
let anchor = AnchorEntity(world: [0,0,-2])
anchor.addChild(entity)
arView.scene.anchors.append(anchor)
}
}
#else
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
}
}
#endif
Deployment target is iOS 10.0:
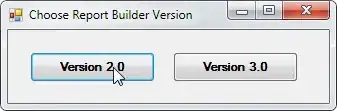
Thirdly :
When publishing to the AppStore (in case we have a deployment target lower than iOS 13.0), we must make the import of this framework weakly linked
in the build settings (that's because RealityKit is deeply integrated in iOS and Xcode).
So, go to Build Settings –> Linking -> Other linker Flags.
Double-click it, press +, and paste the following command:
-weak_framework RealityKit -weak_framework Combine
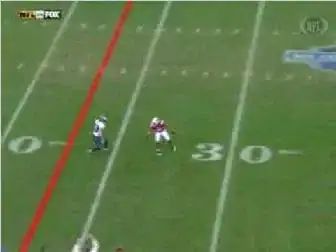
P.S. In Xcode 13.3, there's a project setting that also could help
OTHER_LDFLAGS = -weak_framework RealityFoundation
Fourthly :
So, go to Build Settings –> Framework Search Paths.
Then type there the following command:
$(SRCROOT)
it must be recursive
.
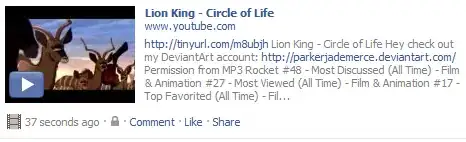
Fifthly
The archives window:
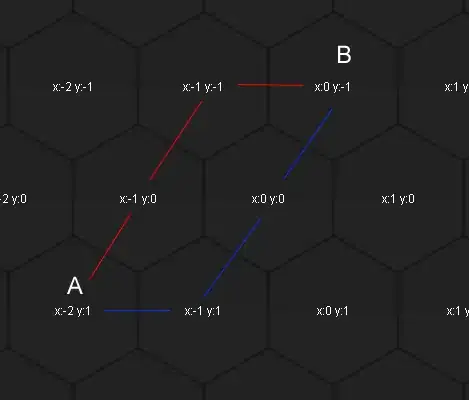