FTP is relatively old and few sites actually still really offer FTP listings most are switching to FTPoverHTTPS:// one example site which is still showing both is
FTP://ftp.gnu.org and it depends how long they may allow simple views such as Windows Explorer, many may only offer an HTML style interface.
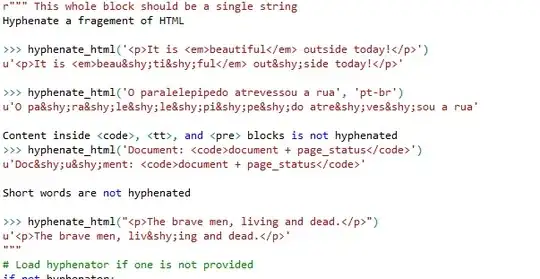
The better ones will include a readme file listing, thus no need to make your own. but if you must you can copy&paste or "save as" site_blahblah.htm
If you need better there are dedicated FTP site browsers, that allow for easy access and listings.
you can for simple sites like above simply write a response file say pdf.txt
user Anonymous
literal pasv
ls *.pdf
bye
and run it via commandline ftp -i -s:pdf.txt -n ftp.gnu.org
the response will be something like
Connected to ftp.gnu.org.
220 GNU FTP server ready.
ftp> user Anonymous
230 Login successful.
ftp> literal pasv
227 Entering Passive Mode (209,51,188,20,108,5).
ftp> ls *.pdf
200 PORT command successful. Consider using PASV.
150 Here comes the directory listing.
blahblah.pdf
blah.pdf
blahblahblah.pdf
226 Directory send OK.
ftp> bye
221 Goodbye.
If you want more details, so for example in place of ls *.pdf lets use real world dir *.gz
and we get:-
ftp> dir *.gz
200 PORT command successful. Consider using PASV.
150 Here comes the directory listing.
-rw-rw-r-- 1 0 3003 240409 Feb 06 21:01 find.txt.gz
-rw-rw-r-- 1 0 3003 462613 Feb 06 21:01 ls-lrRt.txt.gz
-rw-rw-r-- 1 0 3003 543098 Feb 06 21:01 tree.json.gz
226 Directory send OK.
For ad hoc listings its quicker to cut and paste the folder as above. However as pointed out you want to use C# so use the stock listing and work iteratively around that.
using System;
using System.IO;
using System.Net;
namespace Examples.System.Net
{
public class WebRequestGetExample
{
public static void Main ()
{
// Get the object used to communicate with the server.
FtpWebRequest request = (FtpWebRequest)WebRequest.Create("ftp://www.contoso.com/");
request.Method = WebRequestMethods.Ftp.ListDirectoryDetails;
// This example assumes the FTP site uses anonymous logon.
request.Credentials = new NetworkCredential ("anonymous","janeDoe@contoso.com");
FtpWebResponse response = (FtpWebResponse)request.GetResponse();
Stream responseStream = response.GetResponseStream();
StreamReader reader = new StreamReader(responseStream);
Console.WriteLine(reader.ReadToEnd());
Console.WriteLine($"Directory List Complete, status {response.StatusDescription}");
reader.Close();
response.Close();
}
}
}