With M3 androidx.compose.material3.Slider
you can use the thumb
attribute to use a custom thumb.
You can use a simple Spacer
or a Box
to obtain a Rectangle:
var sliderPosition by remember { mutableStateOf(0f) }
val interactionSource = MutableInteractionSource()
Column {
Text(text = sliderPosition.toString())
Slider(
modifier = Modifier.semantics { contentDescription = "Localized Description" },
value = sliderPosition,
onValueChange = { sliderPosition = it },
valueRange = 0f..5f,
steps = 4,
interactionSource = interactionSource,
onValueChangeFinished = {
// launch some business logic update with the state you hold
},
thumb = {
val shape = RectangleShape
Spacer(
modifier = Modifier
.size(20.dp)
.indication(
interactionSource = interactionSource,
indication = rememberRipple(
bounded = false,
radius = 20.dp
)
)
.hoverable(interactionSource = interactionSource)
.shadow(if (enabled) 6.dp else 0.dp, shape, clip = false)
.background(Red, shape)
)
},
)
}
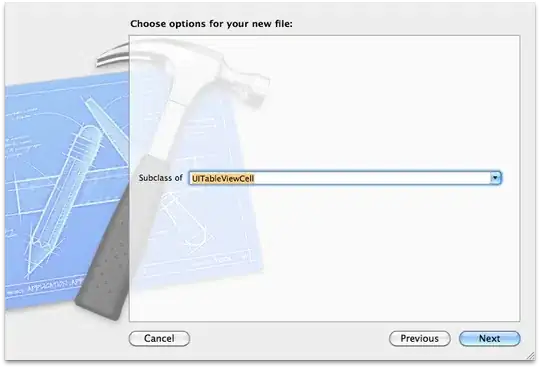
Note: it requires for material3
at least the version 1.0.0-beta03