This is not technically an answer as it uses another package (pyAlluv) that creates matplotlib figures.
The package needs to be installed directly from github, for example with:
pip install --upgrade --no-deps git+https://github.com/tools4digits/pyalluv.git
The example is an adaptation from one of the existing examples:
import numpy as np
from pyalluv import Alluvial
import matplotlib.pyplot as plt
ext = [10, ] # start at t1 with a single node containing 10 elements
flows = [[[5], # flow form node 1 at t1 to node 1 at t2
[2], # flow from node 1 at t1 to node 2 at t2
[3]], # ...
[[4, 0, 0], # flows from nodes 1, 2, 3 at t2 to node 1 at t3
[1, 0, 3]]] # flows from nodes 1, 2, 3 at t2 to node 2 at t3
alluv = Alluvial(x=['t1', 't2', 't3'], flows=flows, ext=ext, width=0.2, yoff=0, layout=['top', 'optimized', 'top'])
plt.show()
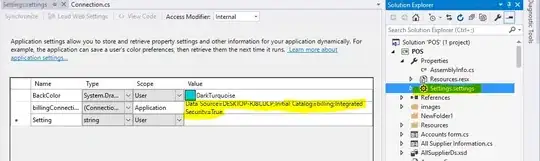
However, note that the package is indicated to be work in progress
It allows for quite some customization, that is not really documented though:
from pyalluv import Alluvial
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ext = [10, ]
flows = [[[5],
[2],
[3]],
[[4, 0, 0],
[1, 0, 3]]]
alluv = Alluvial(ax=ax, x=['t1', 't2', 't3'], flowprops={'fc':'gray', 'alpha':0.4}, ec='gray')
diag0 = alluv.add(flows=flows, ext=ext, width=0.2, yoff=0,
layout=['top', 'optimized', 'top'])
diag0.get_block((1,0)).set_facecolor('red')
diag0.get_block((1,1)).set_facecolor('purple')
diag0.get_block((1,2)).set_facecolor('green')
diag0.get_block((2,0)).set_facecolor('orange')
diag0.get_block((2,1)).set_facecolor('lightblue')
alluv.finish()
plt.show()
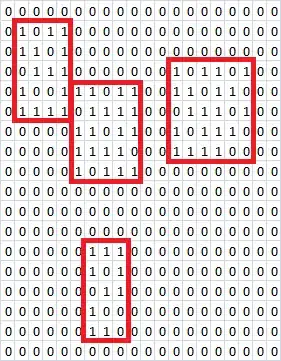