This question is broader than you may think, as there are many ways to deal with it. Here some first ideas:
Let's start with a quick class diagram. The friendship between students is a many-to-many association.
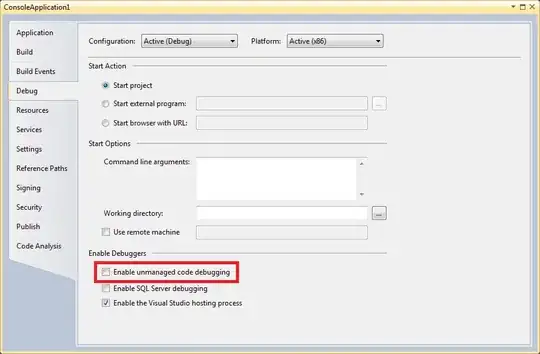
In a database, a many-to-many association is usually implemented using an association table. So you'd have two tables: STUDENTS
and FRIENDSHIPS with pairs of ids of befriended students:
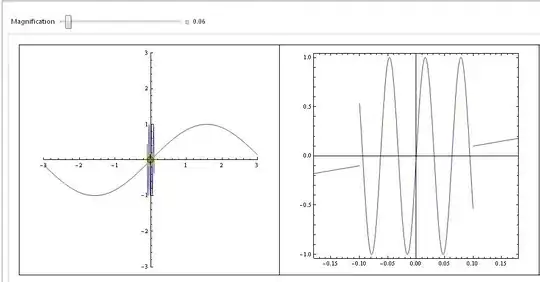
To load a Student
object from the database, you'd read the data in a STUDENTS
row and use it to initialize your object. For the friendship, you'd have to read the relevant FRIENDSHIPS
rows.
But how to use these tables in the application?
- A first possibility would be to load each
Student
friend and insert it in the ArrayList<Student>
. But each loaded student is like the first student and could have oneself friends that you'd have to load as well! You'd end up loading a lots of students, if not all, just for getting the single one you're interested in.
- A second possibility would be use an
ArrayList<StudentId>
instead of an ArrayList<Student>
and populate it. You'd then load the friends just in time, only when needed. But this would require some more important changes in your application.
- A third possibility is not to expose an
ArrayList
. Not leaking the internals is always a good idea. Instead use a getter. So you'd load the friends only if student.getFriends()
is called. This is a convenient approach, as you'd have a collection of friends at your disposal, but avoid being caught in a recursive loading of friends of friends.
In all the cases, you may be interested in using a repository object to get individual or collections of students, and encapsulate the database handling.
Advice: as said, there are many more options, the repository is one approach but there are also active records, table gateways and other approaches. To get a full overview, you may be interested in Martin Fowler's book Patterns of Enterprise Application Architecture.