When you pass a numeric value from Excel to VBA, the value is always passed as Double
, therefore the check for VarType
will not work.
Try the following function: It first checks if the value is numeric at all. If yes, it checks if the integer-part of the number is equal to the number itself. It uses CLng
to avoid numeric overflows.
Function isInteger(c As Variant) As Boolean
If Not IsNumeric(c) Then Exit Function
isInteger = (c = CLng(c))
End Function
Update After freeflow's comment, I did some tests, see image.
It seems that the formatting of a cell doesn't impact the type that is passed to VBA - except if it is a Date. The number 44614
shown as value is in fact the formula =Today()
(so today's date, without time). Only when the cell is formatted as Date, the value is passed as a Date rather than a number.
In all cases, when a number is passed, it is passed as Double.
The column IsInteger
shows the result of the Function above.
(With passed I mean that a variant is passed containing a vartype of...)
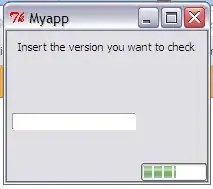