Problem description
How to change the shape of a Row
border in Jetpack Compose?
I'm creating a custom button in jetpack compose and for that I'm using a Row
to align the content horizontally. I need this custom button to have the following format:

However my code in compose renders the following element, without the rounded edges:
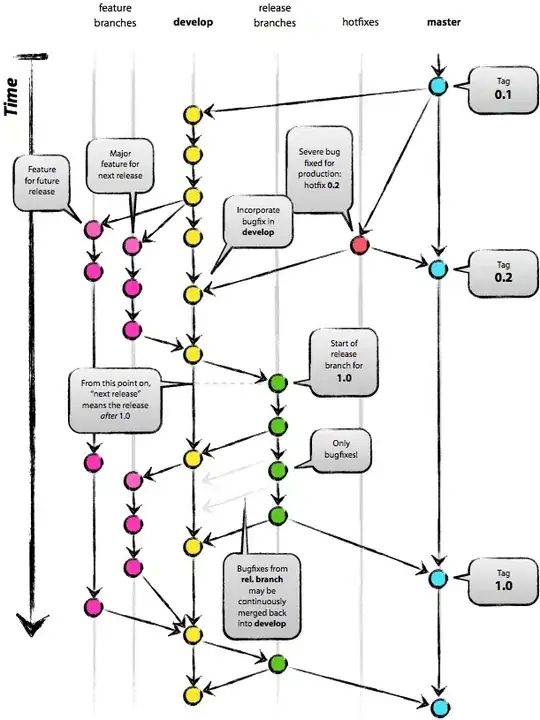
The code for this compose function is below:
@Composable
fun LargeQuantityButton(
modifier: Modifier = Modifier,
onPlusClick: () -> Unit,
onMinusClick: () -> Unit,
text: String,
) = Row(
modifier = modifier
.border(
border = ButtonDefaults.outlinedBorder,
shape = RoundedCornerShape(4.dp)
),
verticalAlignment = Alignment.CenterVertically,
horizontalArrangement = Arrangement.SpaceBetween
) {
RemoveIcon(action = onMinusClick)
Text(
text = text,
fontWeight = FontWeight.W400
)
AddIcon(action = onPlusClick)
}
My attempt fails
I tried adding the clip
function call right after the border
call of the Modifier
like this:
@Composable
fun LargeQuantityButton(
modifier: Modifier = Modifier,
onPlusClick: () -> Unit,
onMinusClick: () -> Unit,
text: String,
) = Row(
modifier = modifier
.border(border = ButtonDefaults.outlinedBorder)
.clip(shape = RoundedCornerShape(4.dp)),
verticalAlignment = Alignment.CenterVertically,
horizontalArrangement = Arrangement.SpaceBetween
) {
RemoveIcon(action = onMinusClick)
Text(
text = text,
fontWeight = FontWeight.W400
)
AddIcon(action = onPlusClick)
}
But it didn't work either.