I think I've worked it out, but I don't have your data. (It's a bit messy.)
I used the dataset diamonds
and renamed the fields. The first plot is supposed to represent your second plot, where you have removed the scale_color_manual
.
The data first:
library(tidyverse)
# create variables
io1 <- diamonds %>%
mutate(year = cut,
effort = x,
clu_name2 = color,
vessel_category = rep(c("Passive","Active"), nrow(diamonds)/2),
geartype_clu2 = rep(LETTERS[1:3], nrow(diamonds)/3))
levels(io1$year) <- c(2019:2023)
Original plot as you've coded:
# grid faceting/color
spaclu <- ggplot(io1, aes(y= effort, x=factor(clu_name2), fill= factor(year))) +
geom_col(position = "dodge") +
theme_minimal()
spaclu + facet_grid(vessel_category~geartype_clu2, scales = "free") +
labs(fill = "Year", x = "Fishing strategies", y = "Total REA",
title = "Based on the REA") +
theme(text = element_text(size = 13)) +
theme(legend.position = "bottom") +
theme(axis.text.x = element_text(angle = 90, vjust = 0.5, hjust=1))
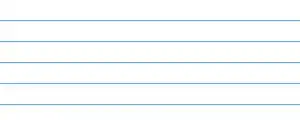
The primary differences are the arguments group = year
and fill = clu_name2
.
p2 <- ggplot(io1, aes(x = clu_name2, y = effort, group = year, fill = clu_name2)) +
geom_col(position = "dodge") +
theme_minimal()
p2 + facet_grid(vessel_category~geartype_clu2, scales = "free") +
labs(fill = "", x = "Fishing strategies\ngrouped by years",
y = "Total REA", title = "Based on the REA") +
theme(text = element_text(size = 13)) +
theme(legend.position = "bottom") +
theme(axis.text.x = element_text(angle = 90, vjust = 0.5, hjust=1))
Keep in mind the legend here is fishing strategies. If you want the years shown, perhaps a second depth label with scale_fill_manual() would be a good approach.

Now as far getting the years and the strategies in the legend or as legends. You may be better off with adding a second scale axis and using the first version. This can be done with the package ggnewscale
. You'll have to adjust the font size or expand or add to the margin, though.
# grid faceting/color
spaclu <- ggplot(io1, aes(y= effort, x=factor(clu_name2), fill= factor(year),
group = year)) +
geom_col(position = "dodge") +
theme_minimal()
spaclu + facet_grid(vessel_category~geartype_clu2, scales = "free") +
labs(fill = "Year", x = "Fishing strategies", y = "Total REA",
title = "Based on the REA") +
theme(text = element_text(size = 13)) +
theme(legend.position = "bottom") +
theme(axis.text.x = element_text(angle = 90, vjust = 0.5, hjust=1)) +
ggnewscale::new_scale("fill") + # added scale here
geom_tile(aes(fill = clu_name2, y = 1)) +
scale_fill_discrete(name = "Strategies")
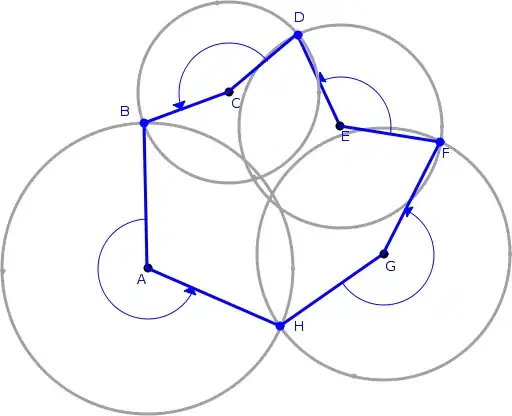
It doesn't quite work out when using it with the second option
p2 <- ggplot(io1, aes(x = clu_name2, y = effort, group = year, fill = clu_name2)) +
geom_col(position = "dodge") +
theme_minimal()
p2 + facet_grid(vessel_category~geartype_clu2, scales = "free") +
labs(fill = "", x = "Fishing strategies\ngrouped by years",
y = "Total REA", title = "Based on the REA") +
theme(text = element_text(size = 13)) +
theme(legend.position = "bottom") +
theme(axis.text.x = element_text(angle = 90, vjust = 0.5, hjust=1)) +
scale_fill_manual(values = c("D" = "#004c6d",
"E" = "#00ffff",
"F" = "#00a1c1",
"G" = "#00cfe3",
"H" = "#78ab63",
"I" = "#6efa75",
"J" = "#ffc334",
"K" = "#ff9509",
"L" = "#ffb6de",
"M" = "#cc0089")) +
ggnewscale::new_scale("fill") +
geom_tile(aes(fill = year, y = 1)) +
scale_fill_viridis_d(name = "Years")
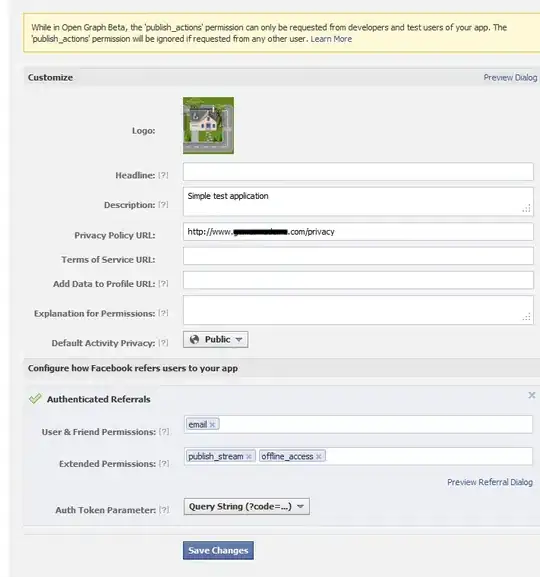