Your strings don't actually have quotes, they just appear that way on the console. Probably it bothers you that you have to set backticks around variable names with spaces, when subsetting with $
.
In R, syntactically valid object names should consist of letters, numbers, dots, and underscores only, no numbers or dots as the first character. You could easily fix that using make.names
.
Example:
df <- data.frame(`big creek`=1:3, `gage creek`=4:6, `garvey creek`=7:9, check.names=F)
df
# big creek gage creek garvey creek
# 1 1 4 7
# 2 2 5 8
# 3 3 6 9
names(df)
# [1] "big creek" "gage creek" "garvey creek" ## invalid names
df$`big creek` ## rather annoying backticks needed
# [1] 1 2 3
cols1 <- names(df) ## vector of column names
cols1
# [1] "big creek" "gage creek" "garvey creek"
make.names(cols1) ## `make.names` fixes names
# [1] "big.creek" "gage.creek" "garvey.creek"
names(df) <- make.names(cols1)
names(df)
# [1] "big.creek" "gage.creek" "garvey.creek" ## valid names
## actually you don't need to cache in a vector and just may do
names(df) <- make.names(names(df))
From then on, use valid names for coding and label your data separately, e.g.:
barplot(colSums(df), names.arg=c("big creek", "gage creek", "garvey creek"))
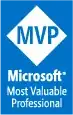
That said, if you still want to have your strings surrounded with backticks, you can use sprintf
.
sprintf('`%s`', cols)
# [1] "`big creek`" "`gage creek`" "`garvey creek`" "`larches creek`" "`little usable`"