Another solution ios the use of the side-by-side bars.
Sample data:
values <- c(21, 22, 33, 21, 27, -71, -77, -67, -79, -73) ##Percentage
sample <- factor(c("Hr", "Fi", "We", "Pa", "Ki"))
mydata <- data.frame(values, sample)
Sample code:
(ggplot(mydata, aes(x = sample, y = values)) +
geom_bar(
stat = "identity", position = position_stack(),
color = "white", fill = "lightblue") +
coord_flip())+
labs(x="Sample",y="Percentage")+
theme_bw()
Plot: 
Plot:
Sample code to show values:
(ggplot(mydata, aes(x = sample, y = values)) +
geom_bar(
stat = "identity", position = position_stack(),
color = "white", fill = "lightblue"
) +
geom_text(aes(label = values))+
coord_flip())+
labs(x="Sample",y="Percentage")+
theme_bw()
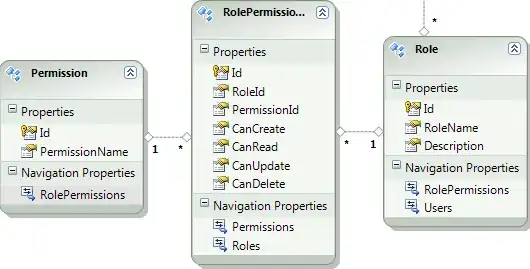
Or if you want to color the positive and negative bars differently
(ggplot(mydata, aes(x = sample, y = values)) +
geom_bar(
stat = "identity", position = position_stack(),
fill= ifelse(mydata$values < 0,"#ffcccb","lightblue")
) +
geom_text(aes(label = values))+
coord_flip())+
labs(x="Sample",y="Percentage")+
theme_bw()
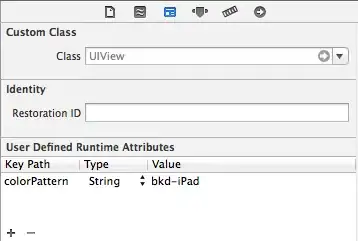