Since you do not provide any reproducible example, I assume that you have a data frame with five columns like this:
DF <- mtcars[,c(1,2,5,6,7)]
head(DF)
# mpg cyl drat wt qsec
#Mazda RX4 21.0 6 3.90 2.620 16.46
#Mazda RX4 Wag 21.0 6 3.90 2.875 17.02
#Datsun 710 22.8 4 3.85 2.320 18.61
#Hornet 4 Drive 21.4 6 3.08 3.215 19.44
#Hornet Sportabout 18.7 8 3.15 3.440 17.02
#Valiant 18.1 6 2.76 3.460 20.22
You want to make one plot that has 5 boxplots, each of which represents each column. It is likely that you want to get this.
boxplot(DF, xlab = "column_names", ylab = "column_values", las = 1)
grid()
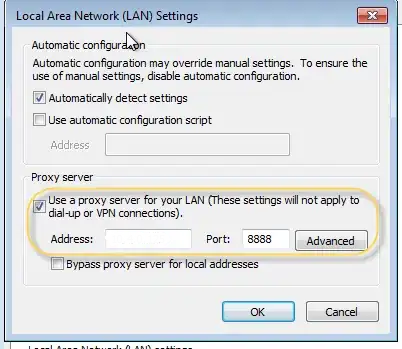
However, you want to get a similar plot by using ggplot. If that's true, you need to transform DF
so that all of the column names of DF
are stored in a single column so that it can be assigned to x
, which ggplot requires. Likewise, all the corresponding values should be stored in another column so that it can be assigned to y
, which ggplot requires. You can do this transformation by using pivot_longer
.
library(tidyverse)
newDF <- DF %>%
pivot_longer(col= everything(),
values_to = "column_values",
names_to = "column_names")
newDF
# A tibble: 160 x 2
# column_names column_values
# <chr> <dbl>
# 1 mpg 21
# 2 cyl 6
# 3 drat 3.9
# 4 wt 2.62
# 5 qsec 16.5
# 6 mpg 21
# 7 cyl 6
# 8 drat 3.9
# 9 wt 2.88
#10 qsec 17.0
# ... with 150 more rows
It is now possible to plot 5 boxplots from newDF
:
newDF %>% ggplot(aes(x = column_names, y = column_values)) +
geom_boxplot(fill = "grey") + theme_bw()
Here is the resulted plot:
