The Swing component JFileChooser
offers getSelectedFile()
and getSelectedFiles()
, which returns an array "of selected files if the file chooser is set to allow multiple selection." In contrast, the JavaFX control FileChooser
returns a single File
from showOpenDialog()
, and it returns a List<File>
from showOpenMultipleDialog()
.
Given a FileChooser
instance, you can obtain the list of selected
files like this:
FileChooser fileChooser = new FileChooser();
List<File> selected = fileChooser.showOpenMultipleDialog(stage);
You can get the first selected
file like this:
System.out.println(selected.get(0));
You can iterate through them like this:
selected.forEach(file -> System.out.println(file.getName() + " selected"));
The complete example below is based on this related example:
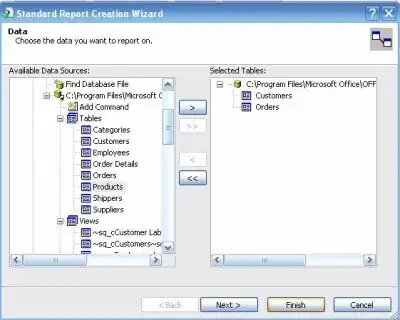
import java.io.File;
import java.util.List;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextArea;
import javafx.scene.layout.VBox;
import javafx.stage.FileChooser;
import javafx.stage.Stage;
/** @see https://stackoverflow.com/a/71362723/230513 */
public class FileChooserDemo extends Application {
private static final int PADDING = 16;
@Override
public void start(Stage stage) {
stage.setTitle("FileChooserDemo");
FileChooser fileChooser = new FileChooser();
Label label = new Label("Select one or more files:");
TextArea textArea = new TextArea();
textArea.setPrefColumnCount(16);
textArea.setPrefRowCount(4);
Button singleButton = new Button("Select a File");
singleButton.setOnAction((ActionEvent t) -> {
File file = fileChooser.showOpenDialog(stage);
if (file != null) {
textArea.clear();
textArea.appendText(file.getName());
}
});
Button multiButton = new Button("Select Files");
multiButton.setOnAction((ActionEvent t) -> {
List<File> selected = fileChooser.showOpenMultipleDialog(stage);
if (selected != null) {
textArea.clear();
selected.forEach(file -> textArea.appendText(file.getName() + "\n"));
}
});
VBox vBox = new VBox(PADDING, label, singleButton, multiButton, textArea);
vBox.setAlignment(Pos.CENTER);
vBox.setPadding(new Insets(PADDING));
Scene scene = new Scene(vBox);
stage.setScene(scene);
stage.show();
}
public static void main(String args[]) {
launch(args);
}
}