You can insert <link rel="prefetch">
elements into the <head>
of the page. This will tell the browser to go ahead and download the thing that it finds in the src
property of that element so that it can be served from the cache if something else on the page (in this case, the audio player) requests it in the future (see docs).
Here's some code that should work:
const alreadyPreloaded = new Set();
export function preloadTrack({ src } = {}) {
// We make sure not to insert duplicate <link rel="prefetch> elements
// in case this function gets called multiple times with the same track.
if (typeof src === "string" && !alreadyPreloaded.has(src)) {
const head = document.getElementsByTagName("HEAD")[0];
const link = document.createElement("link");
link.rel = "prefetch";
link.href = src;
head.appendChild(link);
alreadyPreloaded.add(src);
}
}
Then, in your react component, you could call preloadTrack
as a side-effect of when the track changes:
useEffect(() => {
preloadTrack(tracks[trackIndex]); // Also preload the current track
preloadTrack(tracks[trackIndex + 1]); // tracks[trackIndex + 1] might be undefined, but we wrote the preloadTracks function to safely handle that.
}, [trackIndex]);
See this fork of your codesandbox
You can see that it's working by checking the "network" tab in debug tools. This is what I see on a fresh page reload after clearing the cache, and before clicking on anything.
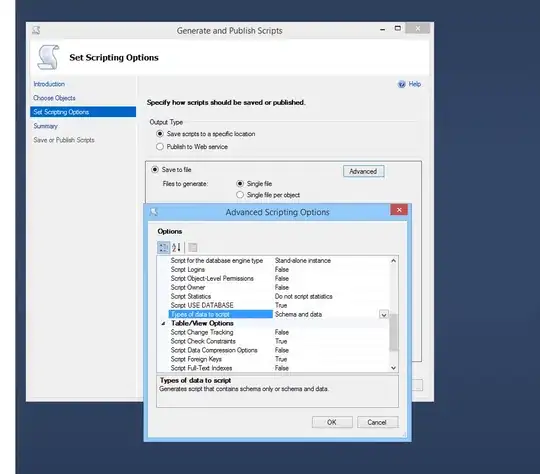
Another way to be sure that it is working, is to hit the "next" button once - you should see that track #2 (bensoud-tenderness.mp3
) is being served from the cache with a 206
status code.
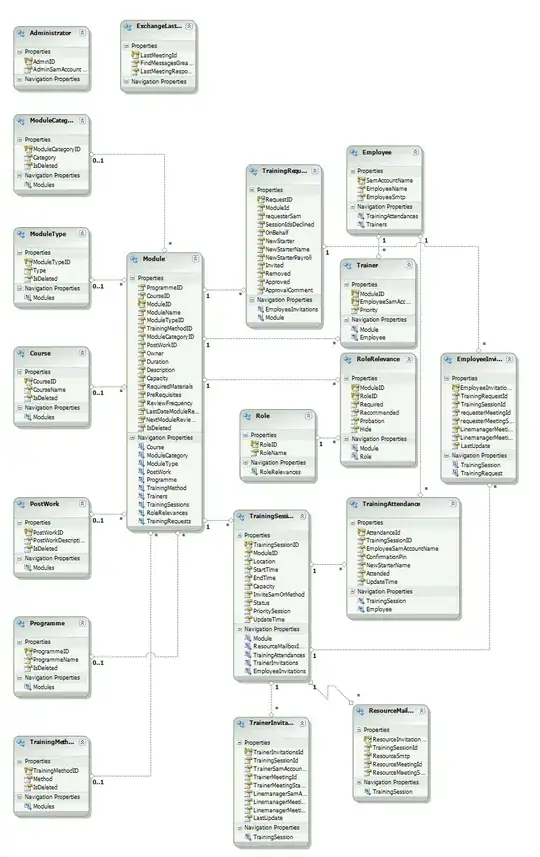