pass it to the static web app on http trigger request
If you want to post it to your Web App You can directly send your JSON content to your web app, here is one of the workaround where you can use Blob trigger and post the data to your web app using HttpWebRequest
. Below is the code that worked for me
using System;
using System.Globalization;
using System.IO;
using System.Text;
using Azure.Storage.Blobs;
using CsvHelper;
using Microsoft.Azure.WebJobs;
using Microsoft.Extensions.Logging;
using Newtonsoft.Json;
using System.Linq;
using System.Threading.Tasks;
using System.Net.Http;
using System.Net;
namespace FunctionApp
{
public static class Function1
{
[FunctionName("Function1")]
public static void Run([BlobTrigger("samples-workitems/{name}", Connection = "AzureWebJobsStorage")]Stream myBlob, string name, ILogger log)
{
log.LogInformation($"C# Blob trigger function Processed blob\n Name:{name} \n Size: {myBlob.Length} Bytes");
var json = Convert(myBlob);
PostJSON(json);
}
public static string Convert(Stream blob)
{
var csv = new CsvReader(new StreamReader(blob), CultureInfo.InvariantCulture);
csv.Read();
csv.ReadHeader();
var csvRecords = csv.GetRecords<object>().ToList();
return JsonConvert.SerializeObject(csvRecords);
}
public static async Task PostJSON(string json)
{
var request = (HttpWebRequest)WebRequest.Create("<Your Web App URL>");
var data = Encoding.ASCII.GetBytes(json);
request.Method = "POST";
request.ContentType = "application/json";
request.ContentLength = data.Length;
using (var stream = request.GetRequestStream())
{
stream.Write(data, 0, data.Length);
}
var response = (HttpWebResponse)request.GetResponse();
var responseString = new StreamReader(response.GetResponseStream()).ReadToEnd();
}
}
}
RESULTS:
Considering this to be my CSV file
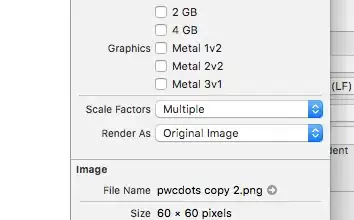
In my selected API
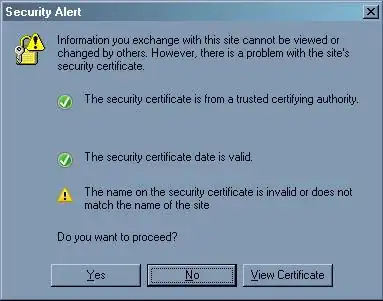
How can I upload the *.CSV file directly from local to be accessible by the function? (to the function directory!?) Where to store the *.json?
You can use blob trigger function in this case, where every time you upload csv file to your storage account it picks the data converts into json file and then stores the same into another container. Please refer the below code for the same
using System;
using System.Globalization;
using System.IO;
using System.Text;
using Azure.Storage.Blobs;
using CsvHelper;
using Microsoft.Azure.WebJobs;
using Microsoft.Extensions.Logging;
using Newtonsoft.Json;
using System.Linq;
namespace FunctionApp
{
public static class Function1
{
[FunctionName("Function1")]
public static void Run([BlobTrigger("samples-workitems/{name}", Connection = "AzureWebJobsStorage")]Stream myBlob, string name, ILogger log)
{
log.LogInformation($"C# Blob trigger function Processed blob\n Name:{name} \n Size: {myBlob.Length} Bytes");
var json = Convert(myBlob);
var fileName = name.Replace(".csv", "");
CreateJSONBlob(json, fileName);
}
public static string Convert(Stream blob)
{
var csv = new CsvReader(new StreamReader(blob), CultureInfo.InvariantCulture);
csv.Read();
csv.ReadHeader();
var csvRecords = csv.GetRecords<object>().ToList();
return JsonConvert.SerializeObject(csvRecords);
}
public static void CreateJSONBlob(string json, string fileName)
{
var c = new BlobContainerClient(Environment.GetEnvironmentVariable("AzureWebJobsStorage"), "jsoncontainer");
byte[] writeArr = Encoding.UTF8.GetBytes(json);
using (MemoryStream stream = new MemoryStream(writeArr))
{
c.UploadBlob($"{fileName}.json", stream);
}
}
}
}
RESULTS:
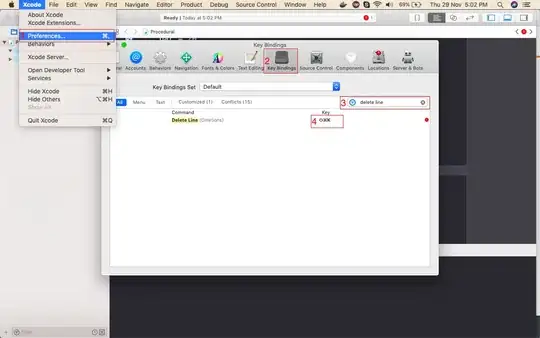
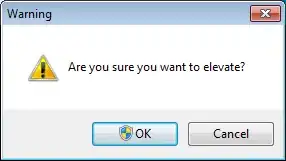
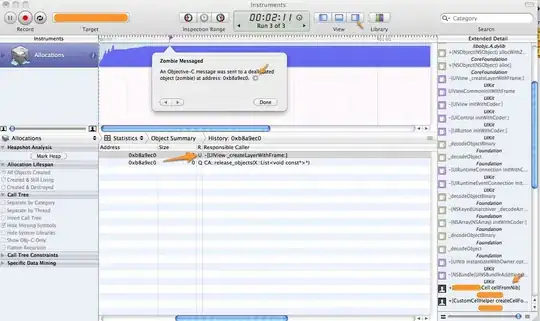
REFERENCES:
Azure Function that converts CSV Files to JSON