Ended up just using standard CSS to implement this, I'll post it here in case anyone wants to utilize (it is modified extensively):
ReactJS/TailwindCSS:
<div id="container" className='dark:bg-[#6052dd] bg-[#aaa0ff] transition ease-out duration-500'>
<div class="item">
<div className='rounded-[30%] shadow-lg w-[100%] py-[2px] h-full bg-gray-200 dark:bg-[#353535] hover:scale-110 duration-500 hover:bg-[#aaa0ff] hover:dark:bg-[#aaa0ff] transition ease-out'>
<img className="w-[90%] my-1 mx-auto" src={images.html} alt="HTML icon" />
</div>
</div>
<div class="item">
<div className='rounded-[30%] w-[100%] shadow-lg py-[1px] h-full bg-gray-200 dark:bg-[#353535] hover:scale-110 duration-500 hover:bg-[#aaa0ff] hover:dark:bg-[#aaa0ff] transition ease-out'>
<img className="w-[90%] my-1 mx-auto" src={images.react} alt="HTML icon" />
</div>
</div>
<div class="item">
<div className='rounded-[30%] w-[100%] shadow-lg py-[1px] h-full bg-gray-200 dark:bg-[#353535] hover:scale-110 duration-500 hover:bg-[#aaa0ff] hover:dark:bg-[#aaa0ff] transition ease-out'>
<img className="w-[90%] my-1 mx-auto" src={images.flutter} alt="HTML icon" />
</div>
</div>
<div class="item">
<div className='rounded-[30%] w-[100%] shadow-lg py-[1px] h-full bg-gray-200 dark:bg-[#353535] hover:scale-110 duration-500 hover:dark:bg-[#aaa0ff] hover:bg-[#aaa0ff] transition ease-out'>
<img className="w-[90%] my-1 mx-auto" src={images.css} alt="HTML icon" />
</div>
</div>
<div class="item">
<div className='rounded-[30%] w-[100%] shadow-lg py-[1px] h-full bg-gray-200 dark:bg-[#353535] hover:scale-110 duration-500 hover:dark:bg-[#aaa0ff] hover:bg-[#aaa0ff] transition ease-out'>
<img className="w-[90%] my-1 mx-auto" src={images.vue} alt="HTML icon" />
</div>
</div>
<div class="item">
<div className='rounded-[30%] w-[100%] shadow-lg py-[0px] h-full bg-gray-200 dark:bg-[#353535] hover:scale-110 duration-500 hover:dark:bg-[#aaa0ff] hover:bg-[#aaa0ff] transition ease-out'>
<img className="w-[90%] my-1 mx-auto" src={images.redux} alt="HTML icon" />
</div>
</div>
<div class="item">
<div className='rounded-[30%] w-[100%] shadow-lg py-[1px] h-full bg-gray-200 dark:bg-[#353535] hover:scale-110 duration-500 hover:bg-[#aaa0ff] hover:dark:bg-[#aaa0ff] transition ease-out'>
<img className="w-[90%] my-1 mx-auto" src={images.firebase} alt="HTML icon" />
</div>
</div>
</div>
The index.css file, this contains the actual transitional animations that achieve the revolving effect:
#container {
--n:7; /* number of item */
--d:45s; /* duration */
width: 500px;
height: 500px;
margin: 40px auto;
display:grid;
grid-template-columns:30px;
grid-template-rows:30px;
place-content: center;
border-radius: 50%;
/* background-color: #aaa0ff; */
}
.item {
grid-area:1/3/3/1;
box-shadow: 50px #000;
line-height: 80px;
text-align: center;
align-self: center;
width: 80px;
height: 80px;
border-radius: 30%;
/* background: rgb(231, 231, 231); */
animation: spin var(--d) linear infinite;
transform:rotate(0) translate(310px) rotate(0);
}
@keyframes spin {
100% {
transform:rotate(1turn) translate(310px) rotate(-1turn);
}
}
.item:nth-child(1) {animation-delay:calc(-0*var(--d)/var(--n))}
.item:nth-child(2) {animation-delay:calc(-1*var(--d)/var(--n))}
.item:nth-child(3) {animation-delay:calc(-2*var(--d)/var(--n))}
.item:nth-child(4) {animation-delay:calc(-3*var(--d)/var(--n))}
.item:nth-child(5) {animation-delay:calc(-4*var(--d)/var(--n))}
.item:nth-child(6) {animation-delay:calc(-5*var(--d)/var(--n))}
.item:nth-child(7) {animation-delay:calc(-6*var(--d)/var(--n))}
/*.item:nth-child(N) {animation-delay:calc(-(N - 1)*var(--d)/var(--n))}*/
What this ends up depicting (this revolves around the center circle, as expected):
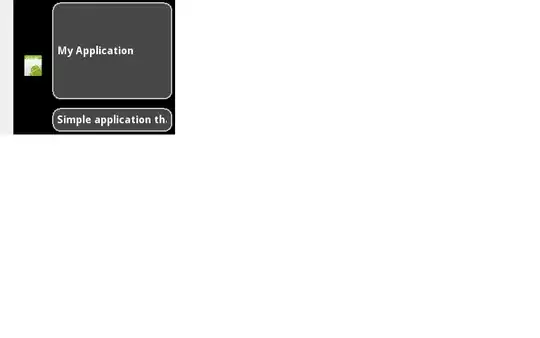
If anyone wants to convert the index.css into TailwindCSS, feel free.