Your raw URLs are not valid. To turn them into valid Google News URLs you want to add row['link'].replace('/./article', '/article')
and add the https://
prefix. Options to obtain the real link, have been discussed here..
This will turn:
news.google.com/./articles/CBMigQFodHRwczovL3d3dy5lc3BuLmNvbS9zb2NjZXIvZ2VybWFuLWJ1bmRlc2xpZ2Evc3RvcnkvNDYzNDMxOC91bmNvbWZvcnRhYmxlLWZyZWlidXJnLWFwcGVhbC1hZnRlci1iYXllcm4tbXVuaWNoLXN1YnN0aXR1dGlvbi1taXgtdXDSAY4BaHR0cHM6Ly93d3cuZXNwbi5jb20vc29jY2VyL2dlcm1hbi1idW5kZXNsaWdhL3N0b3J5LzQ2MzQzMTgvdW5jb21mb3J0YWJsZS1mcmVpYnVyZy1hcHBlYWwtYWZ0ZXItYmF5ZXJuLW11bmljaC1zdWJzdGl0dXRpb24tbWl4LXVwP3BsYXRmb3JtPWFtcA?hl=en-US&gl=US&ceid=US%3Aen
into:
https://news.google.com/articles/CBMigQFodHRwczovL3d3dy5lc3BuLmNvbS9zb2NjZXIvZ2VybWFuLWJ1bmRlc2xpZ2Evc3RvcnkvNDYzNDMxOC91bmNvbWZvcnRhYmxlLWZyZWlidXJnLWFwcGVhbC1hZnRlci1iYXllcm4tbXVuaWNoLXN1YnN0aXR1dGlvbi1taXgtdXDSAY4BaHR0cHM6Ly93d3cuZXNwbi5jb20vc29jY2VyL2dlcm1hbi1idW5kZXNsaWdhL3N0b3J5LzQ2MzQzMTgvdW5jb21mb3J0YWJsZS1mcmVpYnVyZy1hcHBlYWwtYWZ0ZXItYmF5ZXJuLW11bmljaC1zdWJzdGl0dXRpb24tbWl4LXVwP3BsYXRmb3JtPWFtcA?hl=en-US&gl=US&ceid=US%3Aen
To make URLs clickable, you can add the following code, as suggested here:
def make_clickable(val):
return '<a href="{}">{}</a>'.format(val,'To the article')
dfges.style.format({'URL': make_clickable})
Full code:
import pandas as pd
from GoogleNews import GoogleNews
googlenews = GoogleNews()
googlenews.set_encode('utf_8')
googlenews.set_lang('en')
googlenews.set_period('7d')
orte = ["Munich"]
nachrichten = []
for ort in orte:
googlenews.clear()
googlenews.get_news(ort)
table_new = []
for row in googlenews.results():
table_new.append({
'City': ort,
'Title': row['title'],
'Date': row['date'],
'URL': f"https://{row['link'].replace('/./article', '/article')}",
'Source': row['site'], })
df = pd.DataFrame(table_new)
nachrichten.append(df)
dfges = pd.concat(nachrichten, axis='index')
dfges.drop_duplicates(subset=['Title'], keep='last', inplace=True)
print(dfges)
def make_clickable(val):
return '<a href="{}">{}</a>'.format(val,'To the article')
dfges.style.format({'URL': make_clickable})
Output:
Open link in a new tab, which leads to a page redirecting to the original article.
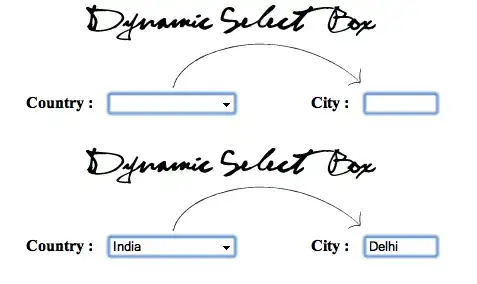