You could use an array's sort(by: )
method.
listOfAnimal.sort { animalOnEarth1, animalOnEarth2 in
// Code... (condition for sort)
// Example: If we want to sort by length of `animals` list.
return animalOnEarth1.animals.count < animalOnEarth2.animals.count
// This will compare each `AnimalOnEarth` object in the array
// (animalOnEarth1) to the one after it (animalOnEarth2).
// If this block returns true, it will sort the first
// (I.e. `animalOnEarth1`) before the next (`animalOnEarth2`).
// If it returns `false`, it will do the opposite.
}
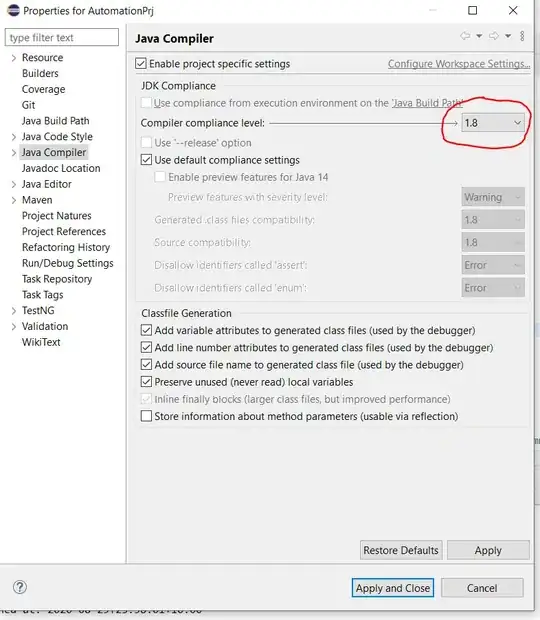
In response to comment:
Thanks for your response, in your solution you are comparing array count though I need to compare values inside the child array to sort parent array - Soniya
It's the same concept. Let's take your image for example, and say we wanted to sort by application fee, lowest to highest.
rowObjectsArray.sort { rowObject1Array, rowObject2Array in
// Get rowObject1Array's application fee. Something like...
var row1ApplicationFee: Int = rowObject1Array.first(where: { $0 is ApplicationFeeObjectName }).applicationFee
// Get rowObject2Array's application fee. Something like...
var row2ApplicationFee: Int = rowObject2Array.first(where: { $0 is ApplicationFeeObjectName }).applicationFee
// Finally, compare...
if row1ApplicationFee < row2ApplicationFee {
return true // meaning we want row1 to stay before row 2
}
else {
return false // meaning we want row1 to actually be after row 2.
}
}